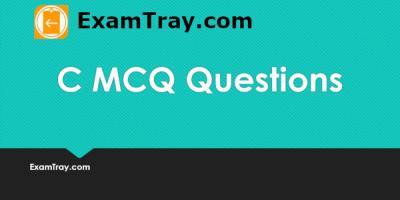
Study C MCQ Questions and Answers on Functions and Pointers. Questions are on Recursion, Pass by Value and Pass By Reference. Attend C technical interviews easily after reading these Multiple Choice Questions.
Go through C Theory Notes on Functions before reading questions.
void texas(int *,int *); int main() { int a=11, b=22; printf("Before=%d %d, ", a, b); texas(&a, &b); printf("After=%d %d", a, b); return 0; } void texas(int *i, int *j) { *i = 55; *j = 65; }
a and b are passed through Call By Reference &a, &b. So the passed addresses are collected by variables i and j. So changes made using pointers are retained even after the function execution is over.
int main() { int a = 66; printf("%d %d %d,\n", a, ++a, a++); a = 66; printf("%d %d %d,\n", ++a, a++, a); a = 66; printf("%d %d %d", ++a, a, a++); return 0; }
68 68 66, 68 66 66, 68 68 66
68 68 66, 66 66 68, 68 68 66
68 68 66, 68 66 68, 68 68 66
68 68 66, 68 66 68, 68 68 68
Order of processing arguments is right to left. First keep 3 placeholder buckets. Right to left check for higher priority of variables like ++a or a++ etc. Always process higher priority variables first. At last, fill the value of low priority variable a.
int main() { int a = 1; printf("%d %d %d,\n", a, ++a, a++); a = 1; printf("%d %d %d,\n", ++a, a++, a); a = 1; printf("%d %d %d", ++a, a, a++); return 0; }
3 3 1, 3 3 3, 3 3 1
3 1 1, 3 1 3, 3 3 1
3 3 1, 3 1 3, 3 1 1
3 3 1, 3 1 3, 3 3 1
void show(int,int,int); int main() { int a = 1; show(++a, a++, a); return 0; } void show(int i, int j, int k) { printf("%d %d %d,\n", i, j, k); }
1 1 3,
3 1 3,
3 1 1,
3 3 3,
1) ++a, 2) a++ 3) a. Second and third arguments have higher priority than third parameter A. So Second is processed. Than first is processed. Finally the actual value of a at that point is given to third place holder.
int main() { int a = 4; int *p; p=&a; while(*p > 0) { printf("%d ", *p); (*p)--; } return 0; }
Notice the decrement operation on variable "a" using (*p)--. Without parantheses, variable is not decremented but memory location pointed is decremented and pointed to a garbage value.
void show(); int show(); int main() { printf("ANT\n"); return 0; } void show() { printf("Integer") ; } int show() { printf("Void"); }
You can not declare same function with different return types. Here both void show() and int show() can not be written.
void show(int); void show(float); int main() { printf("ANT\n"); return 0; } void show(int a) { printf("Integer") ; } void show(float b) { printf("Void"); }
You can not re declare and redefine the a function with same name and different arguments. Function name can not be duplicate in any combination.
int main() { int a=10; int *p, **q; p=&a; q=&p; printf("%d ", a); *p=15; printf("%d ", a); **q=20; printf("%d ", a); return 0; }
Here p is pointer to an integer. q is a pointer to pointer. That is why you should use two STARs **.
int main() { int a=20; int *p, *q; p=&a; q=p; printf("%d ", a); *p=30; printf("%d ", a); *q=40; printf("%d ", a); return 0; }
P is already a pointer to an integer a. Q copied P. So Q is also a pointer to the same integer a.
int main() { int a=20; //a memory location=1234 printf("%d %d %d", a, &a, *(&a)); return 0; }
If VALUEAT operator * and ADDRESSOF operator & are used immediately, it is same as the variable itself. So *(&a) == a.
int main() { int a=20; printf("CINEMA "); return 1; printf("DINOSAUR"); return 1; }
There are two return statements in main() function. Only first return is executed. All the statements or lines of code below first return is not reachable and hence not executed.
int sum(int); int main() { int b; b = sum(4); printf("%d", b); } int sum(int x) { int k=1; if(x<=1) return 1; k = x + sum(x-1); return k; }
4 + 3 + 2 + 1 = 10.
int mul(int); int main() { int b; b = mul(3); printf("%d", b); } int mul(int x) { if(x<=1) return 1; return (x * mul(x-1)); }
3*2*1 = 6.
Yes. Recursion is slow. Variable are kept and remove on STACK memory multiple times.
Yes. To come out of recursion, you must use IF or ELSE blocks.
Recursion is entirely based on RETURN value; statement.
Only one function is required to achieve recursion. Recursion is calling the same function within. How ever to use the value or output of all recursive calls, at least one main() function is also required.