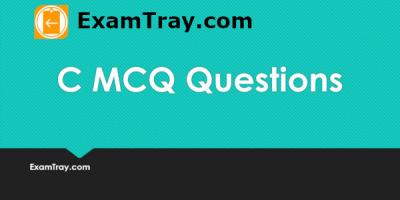
Study C MCQ Questions and Answers on Strings, Character Arrays, String Pointers and Char Pointers. Easily attend technical job interviews after practising the Multiple Choice Questions.
Go through C Theory Notes on Strings before studying questions.
ASCII value of NULL character is ZERO 0.
char ary[] = {'a','b','\0'}.
char bry[] = {`a`,`b`,`c`}; is wrong as it uses Left Single Quotes.
int main() { char var='b'; printf("%d ", sizeof("a")); printf("%d ", sizeof('b')); printf("%d ", sizeof(10)); printf("%d ", sizeof(var)); } //int size is 2 bytes
sizeof('b') is 2 because 'b' is converted to ASCII number which is integer. sizeof(var) is printed as expected. size("a") is two because NULL occupies 1 byte.
int main() { char str[]="JACKIE CHAN"; int i=0; while(str[i] != 0) { printf("%c",str[i]); i++; } return 0; }
Yes. You can check for end of a string with ASCII ZERO 0. ASCII value of NULL or \0 is ZERO.
int main() { char str[]="ANDAMAN"; int i=0; while(str[i] != '\0') { printf("%c",str[i]); i++; } return 0; }
int main() { char str[]="MALDIVES"; printf("%s ",str); puts(str); return 0; }
PUTS prints strings without any format specifier like %s.
int main() { char str[3]="SUNDAY"; printf("%s",str); }
You get C warning.
warning: initializer-string for array of chars is too long
int main() { char str1[]="JAMES,"; char str2[15]="BOND "; strcat(str2,str1); printf("%s",str2); printf("%s",str1); }
Here str1 is not affected by strcat function. STRCAT(destination, source).
int main() { printf("%c","HUMPTY"[2]); }
int main() { char str1[] = "FIRST"; char str2[20]; strcpy(str2,str1); printf("%s %s ",str1,str2); printf("%d", (str1!=str2)); printf("%d", strcmp(str1,str2)); return 0; }
STRCPY copies STR1 value to another memory location pointed by STR2. Only STR1 and STR2 values are same but not memory locations.
int main() { char *code[]={"IN","USA","K"}; printf("%s", code[1]); return 0; }
It is an array of arrays. Using an array of pointers to strings, we can save memory. Different strings can have different lengths. Other wise, max length of any element should be the length of all elements. It wastes space.
int main() { char code[3][4]={"IN","USA","K"}; printf("%s", code[1]); return 0; }
Here, we have not used pointers to strings. So a total of 3x4=12 bytes is allocated. Using a pointers to strings, we can allocate just 3+4+2=9 bytes. Extra byte stores NULL or \0. USA+null = 4 characters.
int main() { char *code[2]; code[0]= (char *)malloc(4); strcpy(code[0], "IND"); printf("%s", code[0]); return 0; }
If you use a pointer to string instead of char str[], you should use malloc() or calloc() to allocate memory and then write some data like "IND" into it. Otherwise, your output will be unexpected.
printf("Hello") takes the address of "Hello" and processes till it reaches NULL or \0.
int main() { char *code="JUMPER"; if(code[6]=='\o') { printf("SUMMER"); } else { printf("WINTER"); } return 0; }
SLASH O is different from SLASH ZERO. Use '\0' to get end of string.