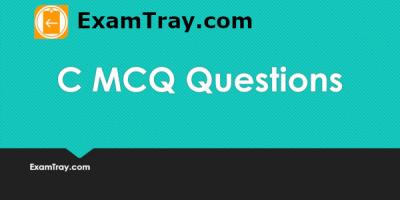
Learn C Data Types and Storage Classes with MCQ Questions and Answers. Find questions on types of Storage Classes like Life, Scope and the default value of variables. Easily attend exams after reading these Multiple Choice Questions.
Go through C Theory Notes on Data Types and Storage Classes before studying questions.
main() { auto int a; printf("%d", a); } //output is compiler error. a is not initialized.
main() { auto int a; printf("%d", a); } //output = 0
main() { auto int a; printf("%d", a); } //output = null
main() { auto int a; printf("%d", a); } //output = some random number
Yes. If an integer is not initialized, some random number in integer range will be displayed.
int main() { printf("Hello Boss."); }
Notice the return type int before main() method. So main should maintain a return 0; or return somenumber; statement.
int main() { auto int a=10; { auto int a = 15; printf("%d ", a); } printf("%d ", a); return 1; }
Automatic or Register variables have block scope and life. Most recent definition takes precedence. {...} is a block.
int main() { register a=10; { register a = 15; printf("%d ", a); } printf("%d ", a); return 20; }
Automatic and Register variables have only Block Scope. Always local definition takes precedence.
register int b; prinf("%d", b);
auto and register variables hold garbage values by default.
int main() { register a=80; auto int b; b=a; printf("%d ", a); printf("%d ", b); return -1; }
void myshow(); int main() { myshow(); myshow(); myshow(); } void myshow() { static int k = 20; printf("%d ", k); k++; }
Variable of type static holds its value until the end of program execution. Static variables do not initialize again and again with function calls.
static int k; int main() { printf("%d", k); return 90; }
Default value of a static variable is zero by default.
int main() { register k = 25; printf("%d", &k); return 90; }
You can not use Ampersand & operator with a Register variable. Register is part of CPU and accessing it is not authorized.
extern int p;
Usually, most of the C statements with extern keyword are Declarations.