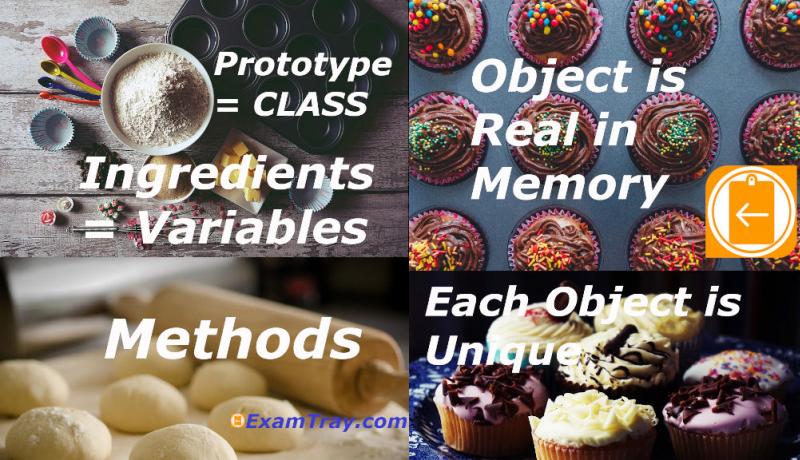
A Java program consists of a CLASS with some Code and Data. Code is also called Method(s). Data is also called Variable(s). Java is an Object-Oriented Language. Let us know more about Java Class Structure and Syntax in this Last Minute Tutorial.
Java Class Structure and Syntax Overview
A Java Class is like a wrapper or envelope that comprises a number of Methods and Variables. In C language, these methods are called Functions. A Java class is like a Blue-Print or Specification-Sheet or Prototype that defines how an Object instantiated out of it (class) to behave and store data.
Parts of a Java Class
A Java Class broadly consists of the following.
- Variables
- Methods
- Constructors
Java Class Syntax
class CLASSNAME { type1 var_1 type2 var_2; type method1(param1,param2) { //code } }
Rules For Creating a Class:
- Each class should contain an Opening Brace "{" and a Closing Brace "}".
- Each method has its own Opening and Closing Braces.
- Each Java class uses the keyword "class" followed by a valid CLASSNAME.
- CLASSNAME or simply "Name" can be any valid identifier.
- CLASSNAME can start with only a Character Alphabet, Underscore (_) or a Dollar ($) sign.
- CLASSNAME can not start with a number or digit. But, numbers may come in the middle or end of the NAME.
- Except Underscore and Dollar, no other special symbols are allowed to be part of an Identifier.
- In a .java file, there can only be one "public class". Other classes may be defined without specifying "public" access specifier.
- There is no restriction on the number of methods present in a class.
- A Java method may or may not return a typed-value.
- A Java method can be public, private, protected or default.
- A Java variable can be public, private, protected or default.
- The MAIN method is not necessary. It is used only to control the project program flow from the start to exit.
- Some projects or frameworks do not allow adding a "main" method to the Java program.
- Some complex Java classes contain "implements" or "extends" keywords next to the CLASSNAME followed by predefined Class-Names. This is part of Inheritance.
Creating a Java Class Object and Using it
A Java object is like a variable of a particular data-type. Here the Object is of the CLASS-type we define. A Class is of no use unless we create Objects. All objects are created at run-time dynamically. Creating an object involves allocating memory to it and returning a reference or handle of the memory location. We create Java objects using a "new" keyword.
A Java Class exists only theoretically or virtually. But an object exists really in memory. A class is comparable to a Structure in a C programming language.
In the above Infographic, we tried to explain what really an Object is. Here, Cupcake Pan is like a prototype. Assume, it has only one mould. It defines what should be the shape of a final Object. Using methods like Kneading, we turned Variables like Ingredients into useful Cupcakes or Objects. Remember that each Object is unique and has its own properties.
An example Java Class is given below. A House object "obj" is created using the "new" keyword. The keyword "new" tells the Java Runtime to allocate some memory to the House object and return a reference to "obj". Now, the object can be accessed using the reference obj.
Each House object has two properties or variables namely width and height. Method getArea() calculates are of the House object and returns it.
public class House { int width, height; int getArea() { return width*height; } public static void main(String[] args) { House obj = new House(); obj.width = 10; obj.height= 20; int area = obj.getArea(); System.out.println("Area of the house = " + area); } } //OUTPUT //Area of the house = 200
Let us know more about Java Classes with different Constructors and Methods in coming chapters.
Share this Last Minute Java Classes and Objects Tutorial with your friends and colleagues to encourage authors.