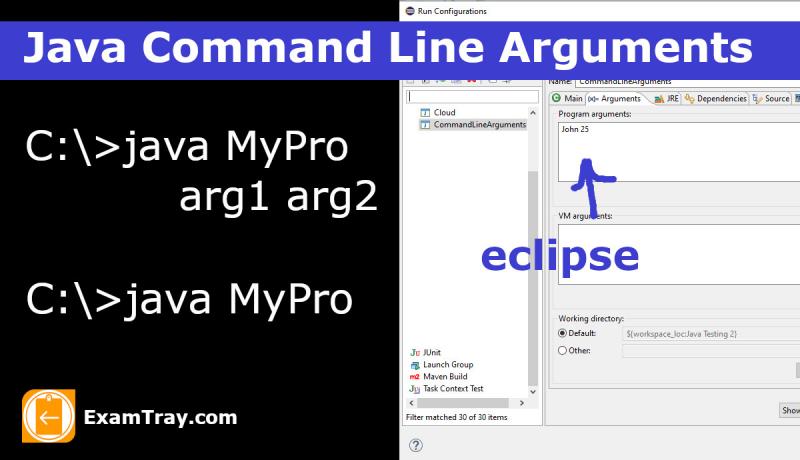
Java command line arguments are nothing but the data passed to the Main method of a Java Class at the time of running the Java Program. This data will be in the form of Strings or a group of characters separated by spaces in general. Let us know more about passing command-line arguments to a Java program using CMD or Eclipse IDE tool.
Read this Java Tutorial on Compiling and Running Java programs using CMD and Eclipse.
Note: You can pass only String values and all Primitive literal values to the Main method. Parsing of data from String to the respective data type like int, float, double, long, char, byte etc is the work of the Java developer. You can parse an "int" value from the String using Integer.parseInt() method for example.
Java Command Line Arguments using CMD
Java Command Line arguments are of String Object type. The number of arguments can range from 0 to any. All command-line arguments are passed on to the MAIN method as a String Array only at Runtime. CMD is our DOS command prompt where we compile and run Java programs.
Let us check the below example that takes Student's details entered as command-line arguments. We have converted String type argument to an int type manually.
public class CommandLineArguments { public static void main(String[] args) { if(args.length >= 2) { String name = args[0]; int age = Integer.parseInt(args[1]); System.out.println("Name= " + name + ", Age= " + age); } else { System.out.println("No arguments passed."); } } }
Now, let us pass arguments to the above Program using CMD or Command-Line like below.
C:\MyPrograms> java CommandLineArguments John 25
The output is as follow.
C:\MyPrograms> Name= John, Age= 25
Now, let us see the output of the program without passing any command line arguments.
C:\MyPrograms> java CommandLineArguments
The output is as follow.
C:\MyPrograms> No arguments passed.
It is a good practice to check the length of the String array "args" inside the Main method, before accessing it. Otherwise, the JVM throws an exception related to Arrays.
Java Command Line Arguments using Eclipse
Eclipse is a Tool to compile, run and manage Java Programs (Projects). It is also called an IDE (Integrated Development Environment). Unlike passing command-line arguments in DOS Prompt, the Eclipse tool provides a GUI screen to enter String arguments. There is no difference in the output whether you use DOS prompt or Eclipse to pass arguments.
Follow the steps to pass Command-Line arguments in Eclipse.
Step 1: Open the Eclipse Tool. Type the Java Program. Save it.
Step 2: Now Click on "Run" in the Menu and then choose "Run Configurations .." option. You will see a Popup.
Step 3: Under the tab "Arguments", enter the arguments separated by spaces. Click the button Run at the bottom of the Popup.
Step 4: Check the Output of Java program under Console in Eclipse.
This is how you can pass command line arguments in Eclipse tool and run a Java program.
Share this Last Minute Java Tutorial on Passing Java Command Line Arguments in CMD and Eclipse with your friends and colleagues.