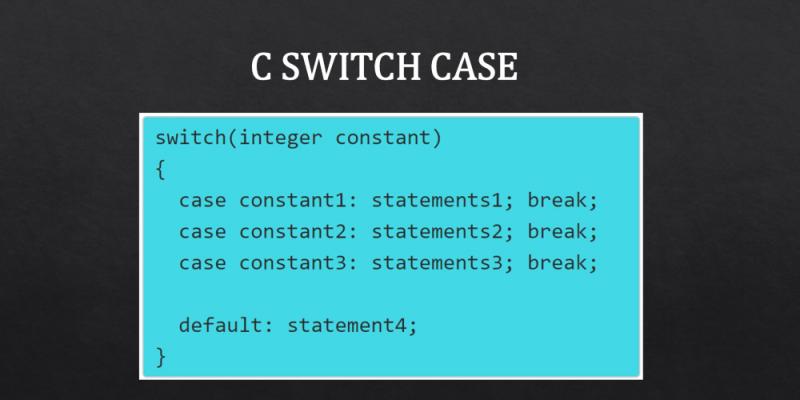
C language provides IF, ELSE IF and ELSE conditional statements to suit various programming needs. Using so many if-else statements look clumsy some times. So, C language an alternative to if-else i.e SWITCH CASE.
Keywords used to implement a SWITCH CASE are switch, case, break and default.
Syntax:
switch(integer constant or expression) { case constant1: statement1; case constant2: statement2; default: statement4; }
Usual way of implementing a SWITCH
switch(integer constant) { case constant1: statements1; break; case constant2: statements2; break; case constant3: statements3; break; default: statement4; }
In the above switch control statement, an integer constant is accepted as a constant. You can use any int data type variable or integer expression as INPUT to a switch. Order of switch implementation is explained below.
Step 1: The input CONSTANT is compared against first case constant i.e constant1. If it matches, corresponding statements are executed.
Step 2: If there is no break; after statements, statements in next case constant section are also executed. There also if there is no break, control goes to the next case constant statements and so on. This way of executing all the statements down the ladder are called SWITCH CASE FALL THROUGH.
Step 3: Finally, if no case constant is matched with input CONSTANT, default case statements are executed. Usually we keep default case at the end of SWITCH. Placement or order of DEFAULT can be anywhere.
Step 4: Switch CASE CONSTANTS can not be Variables.
Step 5: There is no effect of CONTINUE statement in a Switch Statement.
Step 6: Proper use of break statement at the end of statements ensures no side effects in SWITCH implementation.
Example 1:
void display(); int main() { int b=8; display(b); display(10); return 9; } void display(int d) { switch(d) { case 6: printf("SIX");break; case 8: printf("EIGHT"); break; case 9: printf("NINE"); break; default: printf("UNKNOWN");break; } printf("\n"); } //output //EIGHT //UNKNOWN
Above program prints a number in text format using switch case.
Example 2:
int main() { switch('c') { case 'a': printf("A");break; case 'b': printf("B"); break; case 'c': printf("C"); break; default: printf("UNKNOWN");break; } } //output //C
You can character constants inside CASE constants. ASCII values of character constants are nothing but integer numbers.
Online Test on C Switch Case
1. | Switch Case Syntax - Online Test |