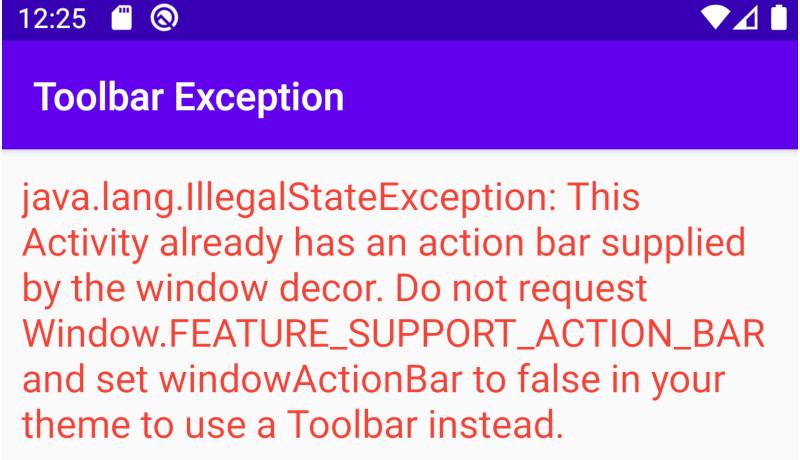
Every Android Activity has a Toolbar or Action-Bar supplied by default by the parent theme "Theme.AppCompat.Light.DarkActionBar". You can find it inside the "styles.xml" file. This theme or style is applied for every Android project created using the available templates. Let us solve this Android exception problem "java.lang.IllegalStateException: This Activity already has an action bar supplied by the window decor. Do not request Window.FEATURE_SUPPORT_ACTION_BAR and set windowActionBar to false in your theme to use a Toolbar instead." easily.
You can also know about how to set the Android Toolbar title at runtime.
How to Solve Android Exception Activity already has an action bar supplied by the window decor
This android exception says that the action-bar or toolbar has been defined already. You are probably adding a more stylized toolbar to the activity which is supplied by either "android.support.v7.widget.Toolbar" or "androidx.appcompat.widget.Toolbar". Follow the below steps to overcome this exception or error.
Step 1: Find styles.xml file under "\app\src\main\res\values".
Step 2: Set the value of the Flag "windowActionBar" to false. Set the value of "windowNoTitle" to true. Give a name for the style such as "AppTheme.NoActionBar". You can simply copy-paste the below XML code in your styles.xml.
<resources> <!-- Base application theme. --> <style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar"> <!-- Customize your theme here. --> <item name="colorPrimary">@color/colorPrimary</item> <item name="colorPrimaryDark">@color/colorPrimaryDark</item> <item name="colorAccent">@color/colorAccent</item> </style> <style name="AppTheme.NoActionBar"> <item name="windowActionBar">false</item> <item name="windowNoTitle">true</item> </style> </resources>
Step 3: Set the Android theme as "AppTheme.NoActionBar" in your activity under AndroidManifest.xml. This removes the default ActionBar or Toolbar and makes the way open for a custom Toolbar. Specify this theme property to each and every <activity> tag separately. The sample code is below.
<application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="Testing2" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity" android:label="Activity Title" android:theme="@style/AppTheme.NoActionBar"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application>
Step 4: Now, you can happily set a SupportActionBar in your MainActivity.java like below. You will not get any Android exception or error about the Action Bar. You can find the Toolbar-XML code here under the activity_main.xml.
import androidx.appcompat.app.ActionBar; import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import androidx.appcompat.widget.Toolbar; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Toolbar toolbar = (Toolbar)findViewById(R.id.toolbar); setSupportActionBar(toolbar); ActionBar actionBar = getSupportActionBar(); if(actionBar != null) { actionBar.setTitle("Custom Toolbar"); } } }
This is how we can ignore calling Window.FEATURE_SUPPORT_ACTION_BAR and set windowActionBar to false to avoid the Toolbar exception using the above Android tutorial.
Learn Java basics before diving into Android for fast coding and development.
You can Share this Android Tutorial with your friends and colleagues to encourage authors.