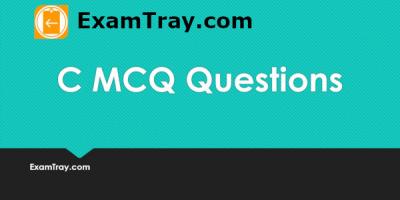
Study C MCQ Questions and Answers on Functions and Pointers. Questions are on Recursion, Pass by Value and Pass By Reference. Attend C technical interviews easily after reading these Multiple Choice Questions.
Go through C Theory Notes on Functions before reading questions.
main() { printf("Hello"); }
int main() { void show() { printf("HIDE"); } show(); return 0; }
Notice that show() function is defined inside main() function. It will not produce a compile error. But, it is not recommended to define a FUNCTION INSIDE A FUNCTION. DO NOT DO.
void show(); int main() { show(); printf("ARGENTINA "); return 0; } void show() { printf("AFRICA "); }
First show() function is called. So it prints AFRICA first.
int main() { show(); printf("BANK "); return 0; } void show() { printf("CURRENCY "); }
Yes. Compiler error. Before calling the show(); function, its Function Prototype should be declared before outside of main() and before main().
void show(); int main() { show(); printf("BANK "); return 0; }
Using a return val; statement, you can return only one value.
void show(); void main() { show(); printf("RAINBOW "); return; } void show() { printf("COLOURS "); }
VOID functions should not return anything. RETURN; is returning nothing.
1. First void main() return; nothing. Still it is valid.
2. Second void show() function is NO RETURN statement. It is also valid.
void show(); void main() { printf("PISTA "); show(); } void show() { printf("CACHEW "); return 10; }
void show() function should not return anything. So return 10; is not recommended.
int show(); void main() { int a; printf("PISTA COUNT="); a=show(); printf("%d", a); } int show() { return 10; }
int show() function returns TEN (10). 10 is assigned to a at a=show().
void main() { int a; printf("TIGER COUNT="); a=show(); printf("%d", a); } int show() { return 15; return 35; }
More than one return statement will not cause Compiler Error. But only FIRST return STATEMENT is executed. Anything after return 15; is not reachable.
int show(); void main() { int a; a=show(); printf("%d", a); } int show() { return 15.5; return 35; }
It is perfectly Okay to return a float number 15.5 as an Integer inside int show() function. 15.5 is demoted to integer as 15 and returned.
int myshow(int); void main() { myshow(5); myshow(10); } int myshow(int b) { printf("Received %d, ", b); }
Notice the function prototype declaration int myshow(int). If you declare wrong either Compiler warning or error is thrown. myshow(5) passes number 5. 5 is received as variable int b.
int myshow(int); void main() { int a=10; myshow(a); myshow(&a); } int myshow(int b) { printf("Received %d, ", b); }
a is 10. &a is the address of the variable a which is a random memory location. To receive an address, int myshow(int b) should be rewritten as int myshow(int *k).
int myshow(int *); void main() { int a=10; myshow(&a); } int myshow(int *k) { printf("Received %d, ", *k); }
It is called Passing a variable by reference. You are passing &a instead of a. Address of a or &a is received as int *k. Observe the function prototype declaration before main(), int myshow(int *).
void myshow(int *); void main() { int a=10; printf("%d ", a); myshow(&a); printf("%d", a); } void myshow(int *k) { *k=20; }
You passed &a instead of a into myshow(int) function. *k=20 changes the valued of passed variable passed by reference.
void myshow(int); void main() { int a=10; printf("%d ", a); myshow(a); printf("%d", a); } void myshow(int k) { k=20; }
You passed variable a directly by value. myshow(a). k=20 will not actually change the variable a as variable k and variable a are completely different. It is called Pass By Value.