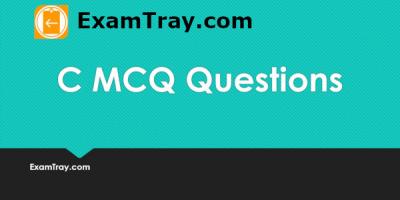
Learn C Programming MCQ Questions and Answers on Loops like While Loop, For Loop and Do While Loop. Loops execute a series of statements until a condition is met or satisfied. Easily attend exams after reading these Multiple Choice Questions.
Go through C Theory Notes on Loops before studying questions.
while(condition) { //statements }
{ //statements }while(condition)
while(condition); { //statements }
while() { if(condition) { //statements } }
for(initalization; condition; incrementoperation) { //statements }
for(declaration; condition; incrementoperation) { //statements }
for(declaration; incrementoperation; condition) { //statements }
for(initalization; condition; incrementoperation;) { //statements }
increment or decrement operation at third place.
dowhile(condition) { //statements }
do while(condition) { //statements }
do { //statements }while(condition)
do { //statements }while(condition);
Semicolon after while(condition) is a must.
int main() { while(true) { printf("RABBIT"); break; } return 0; }
while(TRUE) or while(true) does not work. true is not a keyword.
int main() { int a=5; while(a==5) { printf("RABBIT"); break; } return 0; }
If there is no BREAK statement, while loop runs continuously util the computer hangs. BREAK causes the loop to break once and the statement below the while if any will be executed.
int main() { int a=5; while(a=123) { printf("RABBIT\n"); break; } printf("GREEN"); return 0; }
while(a=123) = while(123) = while(Non Zero Number). So while is executed. BREAK breaks the loop immediately. Without break statement, while loop runs infinite number of times.
int main() { int a=5; while(a >= 3); { printf("RABBIT\n"); break; } printf("GREEN"); return 0; }
Notice a semicon(;) after while condition. It makes the printf and break statement blocks isolate.
while(a >= 3) { ;//infinite loop } { printf("RABBIT\n"); break; }
int main() { int a=25; while(a <= 27) { printf("%d ", a); a++; } return 0; }
a++ is equivalent to a=a+1;
a is incremented each time.
int main() { int a=32; do { printf("%d ", a); a++; }while(a <= 30); return 0; }
do { } block is executed even before checking while(condition) at least once. This prints 32. To loop for the second time, while (32 <= 30) fails. So, loop is quit.
int main() { int a=32; do { printf("%d ", a); a++; if(a > 35) break; }while(1); return 0; }
while(1) is infinite loop. So we kept if(condition) to break the loop. a++ is equivalent to a=a+1;
int main() { int k, j; for(k=1, j=10; k <= 5; k++) { printf("%d ", (k+j)); } return 0; }
You can initialize any number of variables inside for loop.
int main() { int k; for(k=1; k <= 5; k++); { printf("%d ", k); } return 0; }
Semicolon at the end of for(); isolates the below print() block. After for loop is over, k value is 6.
for(k=1; k <= 5; k++) { ; } { printf("%d ", k); }
int main() { int k; for(;;) { printf("TESTING\n"); break; } return 0; }
for(;;) loop need not contain any initialization, condition and incre/decrement sections. All are optional. BREAK breaks the FOR Loop.
int main() { int k; for(printf("FLOWER "); printf("YELLOW "); printf("FRUITS ")) { break; } return 0; }
for(anything; anything; anything) is Ok. printf("YELLOW") prints YELLOW and returns 1 as result. So for loop runs forever. Actually break is saving us from quitting the for loop. Only after checking condition and executing the loop statements, third section is executed. break causes the loop to quit without incre/decrement section.
eg. while(condition) { break; }