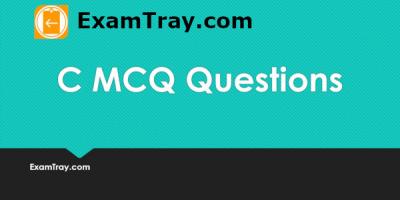
Study C MCQ Questions and Answers on Structures and Pointers. C Structures are widely used in the code of hardware drivers and operating systems. Easily attend technical job interviews after reading these Multiple Choice Questions.
Go through C Theory Notes on Structures and Pointers before studying these questions.
struct insurance { int age; char name[20]; }
Individually calculate the sizes of each member of a structure and make a total to get Full size of a structure.
int main() { structure hotel { int items; char name[10]; }a; strcpy(a.name, "TAJ"); a.items=10; printf("%s", a.name); return 0; }
Keyword used to declare a structure is STRUCT not structURE in lowercase i.e struct.
int main() { struct book { int pages; char name[10]; }a; a.pages=10; strcpy(a.name,"Cbasics"); printf("%s=%d", a.name,a.pages); return 0; }
pages and name are structure members. a is a structure variable. To refer structure members use a DOT operator say a.name.
struct book { int SNO=10; //not allowed };
int main() { struct ship { }; return 0; }
int main() { struct ship { int size; char color[10]; }boat1, boat2; boat1.size=10; boat2 = boat1; printf("boat2=%d",boat2.size); return 0; }
Yes, it is allowed to assign one structure variables. boat2=boat1. Remember, boat1 and boat2 have different memory locations.
int main() { struct ship { char color[10]; }boat1, boat2; strcpy(boat1.color,"RED"); printf("%s ",boat1.color); boat2 = boat1; strcpy(boat2.color,"YELLOW"); printf("%s",boat1.color); return 0; }
boat2=boat1 copies only values to boat2 memory locations. So changing boat2 color does not change boat1 color.
int main() { struct tree { int h; } struct tree tree1; tree1.h=10; printf("Height=%d",tree1.h); return 0; }
Notice a missing semicolon at the end of structure definition.
struct tree { int h; };
int main() { struct tree { int h; int w; }; struct tree tree1={10}; printf("%d ",tree1.w); printf("%d",tree1.h); return 0; }
struct tree tree1={10};
Assigns the value to corresponding member. Remaining are set to Zero. So w is zero.
int main() { struct tree { int h; int rate; }; struct tree tree1={0}; printf("%d ",tree1.rate); printf("%d",tree1.h); return 0; }
Easiest way of initializing all structure elements.
struct tree tree1={0};
int main() { struct laptop { int cost; char brand[10]; }; struct laptop L1={5000,"ACER"}; struct laptop L2={6000,"IBM"}; printf("Name=%s",L1.brand); return 0; }
You can initialize structure members at the time of creation of Structure variables.
int main() { struct forest { int trees; int animals; }F1,*F2; F1.trees=1000; F1.animals=20; F2=&F1; printf("%d ",F2.animals); return 0; }
F2.animal is not allowed as F2 is a pointer to structure variable. So use ARROW operators. F2->animal.
int main() { struct bus { int seats; }F1, *F2; F1.seats=20; F2=&F1; F2->seats=15; printf("%d ",F1.seats); return 0; }
DOT operator is used with a structure variable. ARROW operator is used with a pointer to structure variable.
int main() { struct pens { int color; }p1[2]; struct pens p2[3]; p1[0].color=5; p1[1].color=9; printf("%d ",p1[0].color); printf("%d",p1[1].color); return 0; }
You can declare and use structure variable arrays.
int main() { struct car { int km; }*p1[2]; struct car c1={1234}; p1[0]=&c1; printf("%d ",p1[0]->km); return 0; }
It is allowed to create array of pointers to structure variables.