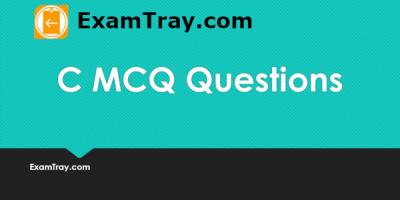
Learn C Programming MCQ Questions and Answers on Conditional Statements like Ternary Operator, IF, ELSE and ELSE IF statements. Easily attend exams after reading these Multiple Choice Questions.
Go through C Theory Notes on Conditional Operators before studying questions.
int main() { int x=1; float y = 1.0; if(x == y) { printf("Polo\n"); } if( 1 == 1.0) { printf("Golf\n"); } if( 1.0 == 1.0f ) { printf("Boxing\n"); } return 0; }
Integer is promoted to float or double automatically before comparison. So all are equal.
1 == 1.0 == 1.0f
int main() { int a=9; if(a=8) { printf("Kangaroo\n"); } printf("Eggs\n"); return 0; }
a=8 is an assignment not comparison. IF( Non Zero) is always TRUE.
int main() { int a=9; if(a==5); { printf("Kangaroo\n"); } printf("Eggs\n"); return 0; }
Notice a Semicolon at the end of IF(a==5);. So IF block ends without any statements. Also, { } is not part of IF now. So it is executed without checking for any condition.
int main() { int a=9; if(a==9); { printf("Ostrich\n"); } elseif(a==8) { printf("Eggs\n"); } printf("White"); return 0; }
Notice IFELSE statement. There should be one SPACE between IF and ELSE.
int main() { int a=9; if(a==9) { printf("Ostrich\n"); } else; { printf("Eggs\n"); } printf("White"); return 0; }
Notice a Semicolon (;) at the end of ELSE. So ELSE is terminated immediately. Eggs Printf block is not part of ELSE and is always called.
int main() { int a=9, b=5, c=8; a=b=c=10; if(a==9) { printf("Ostrich\n"); } else { printf("Eggs\n"); } printf("White"); return 0; }
int main() { int a=9, b=5, c=8; if(!(a==9)) { printf("Bear\n"); } else { printf("Elephant\n"); } printf("Fox"); return 0; }
Logical Not Operator ( ! ) changes true to false and false to true. So IF(false) is not executed.
Logical NOT Operator in C has the highest priority.
int main() { int a=9, b; b = (a==9) ? (printf("CAT\n");printf("DOG\n")) : (printf("FOX")); return 0; }
You can not put more than 1 statement inside expression1 or expression2 inside Ternary Operator.
(Condition)?(expression1):(expression2)
int main() { int a=9, b=6; if(a==9 && b==6) { printf("Hockey"); } else { printf("Cricket"); } return 0; }
== operator has more priority than &&. Logical && AND operator returns true only if both expressions are true.
int main() { int a=9, b=6; if(a!=9 || b==6) { printf("Hockey\n"); } else { printf("Cricket\n"); } printf("Football"); return 0; }
Logical OR || operator returns true if any one expression is true.
( ! Logical NOT ) > (*, /, % Arithmetic) > (+, - Arithmetic) > ( <, <=, >, >= Relational) > (==, != Relational) > (&& Logical AND) > (|| Logical OR) > (= Assignment).
int main() { int a=5, b=8; if( a==5 && (b=9) ) { printf("Gorilla Glass="); } printf("%d %d", a, b); return 0; }
In IF( a==5 && (b=9) ), && Operator checks both expressions for true or Non Zero value. So after checking a==5, b=9 is checked. Here b==9 is checking, but b=9 is assignment. Any NON-Zero value is true only. So b=9 now.
int main() { int a=5, b=8; if( a==5 || (b=9) ) { printf("Gorilla Glass="); } printf("%d %d", a, b); return 0; }
Logical OR || checks wants only one TRUE condition. First expression (a==5) or even (a=5) is true. So second expression (b=9) is not evaluated and assignment not done. So b=8 only.
int main() { int a=5, b=8; if( a>=5 || (b=9) ) { printf("Gorilla Glass"); } return 0; } //OUTPUT: Gorilla Glass
int main() { int a=5, b=8; if( a >= 5 && b <= 9 ) { printf("Gorilla Glass"); } return 0; } //OUTPUT: Gorilla Glass