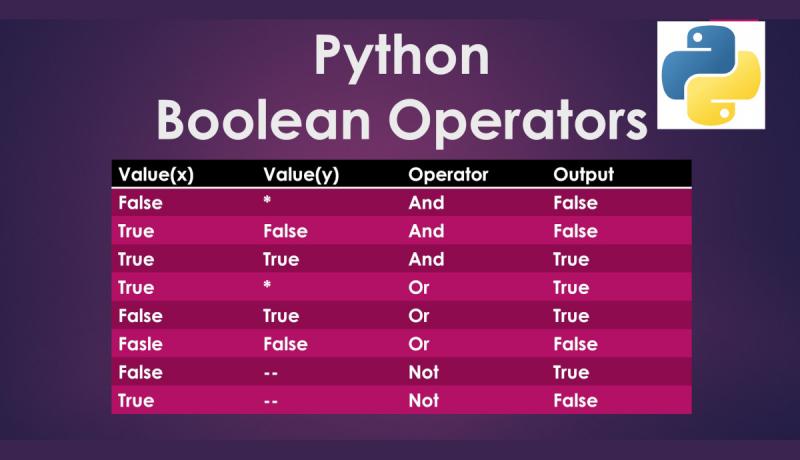
Python uses the same keywords to represent logical operators and boolean operators. These are "and", "or" and "not". Let us learn more in this Last Minute Python Boolean Operators and Priority Tutorial with examples.
Note: Python boolean operators are keywords but not symbols.
Python Boolean Operators
If boolean operands are used along with "and", "or" or "not", the operation is called boolean operation. The operators are called boolean-operators. The output of boolean operations is of bool data type, either True or False. If non-boolean operands are used, those operators are called logical-operators.
Boolean operators are used to combining multiple conditions similar to logical operators. Each individual condition may be built-up of arithmetic or relational operations or both.
1. Python AND Boolean Operator
This operator is also called Short Circuit Boolean AND. It gives the output as True only if both the operands are True. If the first operand is False, it does not evaluate the second operand. It gives False as the output directly.
Boolean AND Truth Table:
Value1 | Value2 | Output |
False | False | False |
False | True | False |
True | False | False |
True | False | False |
Example:
a=False; b=True; c=10 if(b and c): print('CEMENT'); print(b and c); print(a and b); #output #CEMENT #10 #False if(c and b): print(c and b) #output #True
2. Python OR Boolean Operator
This operator is also called Short Circuit Boolean OR. It gives the output as True if the any of the operands are True. If the first operand is True, this Boolean OR operator does not evaluate the second operand. It gives the output as True directly.
Boolean OR Truth Table:
Value1 | Value2 | Output |
False | False | False |
False | True | True |
True | False | True |
True | True | True |
Example:
p=True; k=False; g=20; if(p or k): print('SAND'); print(p or k); print(k or g); #output #SAND #True #20 if(k or g): print(k or g) print(g or k) print(k or p) #output #20 #20 #True
3. Python NOT Boolean Operator
This operator gives the output as False if the input is True. If the input is False, it gives True as the output. So it reverses the input.
Boolean NOT Truth Table:
Input | Output |
False | True |
True | False |
Example:
p=True; k=False; if(not k): print("NOT K") print(not k) #output #NOT K #True print(not p) #output #False
Python Boolean Operator Priority or Precedence
The boolean NOT has the highest priority among all 3 boolean operators. The boolean AND has the least priority.
Python Boolean operators have the same priority as that of Logical operators. Also, boolean operators have less priority when compared to Unary, Arithmetic, Bitwise, Relational and Assignment operators.
Boolean operator priority table:
Operator | Name | Priority |
not | Boolean NOT | 1 |
or | Boolean OR | 2 |
and | Boolean AND | 3 |
In the coming chapters, you will know how to use Python Bitwise operators.
Share this Last Minute Python tutorial on Boolean Operators and their Priority with your friends and colleagues to encourage authors.