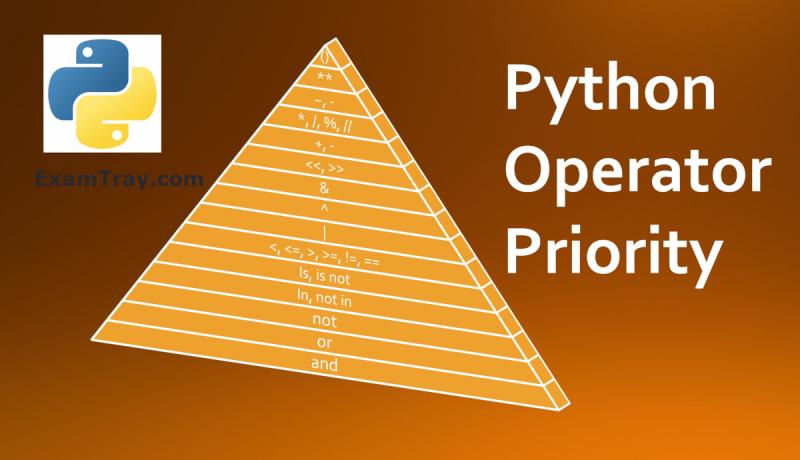
Python programming language supports a variety of operators namely Arithmetic, Bitwise, Relational, Assignment, Identity, Membership, Logical and Boolean. Let us discuss Python Operator Priority or precedence relative to one other with examples using this tutorial.
The Python operator associativity is to know or decide to which operator, an operand belongs to. Usually, Left to Right associativity is applied in Python meaning that an operand belongs to the operator next to it. But before applying the associativity, an operator's priority must be resolved. Check the examples given in this tutorial to learn operator associativity.
Python Operator Priority or precedence
Each operator in a specific operator group may have different priority. With respect to one operator-group, other operator-group can have different priority.
You need to remember these priorities so that you can better write and evaluate expressions involving a variety of operators.
Parentheses operator has got the highest priority among all operators. Parentheses are used to group small expressions or conditions. The Exponential operator (**) stands second in the overall priority table. In the third position, Bitwise Complement (~) and Unary Minus (-) sit.
The logical and boolean operators have the least priority. These two operator types share the same keywords to represent operators.
Just remember that the operator-groups according to their priority are Arithmetic, Bitwise, Relational, Assignment, Identity, Membership, Logical and Boolean.
Python Operator Priority table is as below.
Operator | Priority |
Parentheses () | 1 |
Exponential (**) | 2 |
Bitwise Complement (~), Unary Minus (-) | 3 |
Arithmetic: Multiplication (*), Division (/), Modulo Divison (%), Integer Division (//) | 4 |
Arithmetic: Addition (+), Subtraction (-) | 5 |
Bitwise Left Shift (<<), Bitwise Right Shift (>>) | 6 |
Bitwise AND (&) | 7 |
Bitwise Exclusive OR (^) | 8 |
Bitwise OR (|) | 9 |
Relational: Less Than (<), Less Than or Equal To (<=), Greater Than (>), Greater Than or Equal To (>=), Equals To (=), Not Equal To (!=) | 10 |
Assignment & Compound-Assignment Operators: =, +=, -=, *=, /=, %=, //=, **= | 11 |
Identity Operators: is, is not | 12 |
Membership Operators: in, not in | 13 |
Logical or Boolean: not | 14 |
Logical or Boolean: or | 15 |
Logical or Boolean: and | 16 |
Example Program 1: In the below example, the exponential-operator (**) has higher priority than multiplication-operator (*). So, b is associated with ** operator. (b**2) is associated with * operator. Addition operator (+) has a lower priority than the multiplication operator.
a=10; b=3; c=2; d = a+b**2*c print('d=', d) #output #10+3**2*2 #10+9*2 #exponetial ** #10+18 #multiplication * #28 #addition #= assignment to variable d
Example Program 2:In the below example, you can see Arithmetic Operators, Bitwise Operators and Relational Operators.
Multiplication-operator (*) has a higher priority than Less-Than-Or-Equal-To operator (<=) and Bitwise-Left-Shift operator (<<). Next, Bitwise-Left-Shift has higher priority than the Relational operator. Variable x is associated with * operator. Variable y is associated with << operator. (x*2) is associated with <= operator.
x=20; y=10; print(y, '=', bin(y)) print('y<<1=', bin(y<<1), '(', y<<1 ,')') if(x*2<=y<<1): print("PRINTER") else: print("PAPER") #output #10 = 0b1010 #y<<1= 0b10100 ( 20 ) #PAPER #order of evaluation #x*2<=y<<1 #20*2<=10<<1 #40<=10<<1 #40<=20 #False
Example Program 3: In the below example, you can see the operator groups like Arithmetic, Compound Assignment and Exponential.
Exponential operator (**) has a higher priority than other operators in the expression. Next comes the Arithmetic operator (*). After the addition (+), finally, the Compound-Assignment operator (+=) is applied. Variable n is associated with ** operator. Variable k is associated with * operator. (k*3) is associated with + operator.
p=10; k=30; n=2; p+=k*3+n**3 print(p) #output #108 #order of evaluation #p+=k*3+n**3 #p+=30*3+2**3 #p+=30*3+8 #p+=90+8 #p=p+98 #108 #p=108
Share this Last Minute Python tutorial on Python Operator Priority with your friends and colleagues to encourage authors.