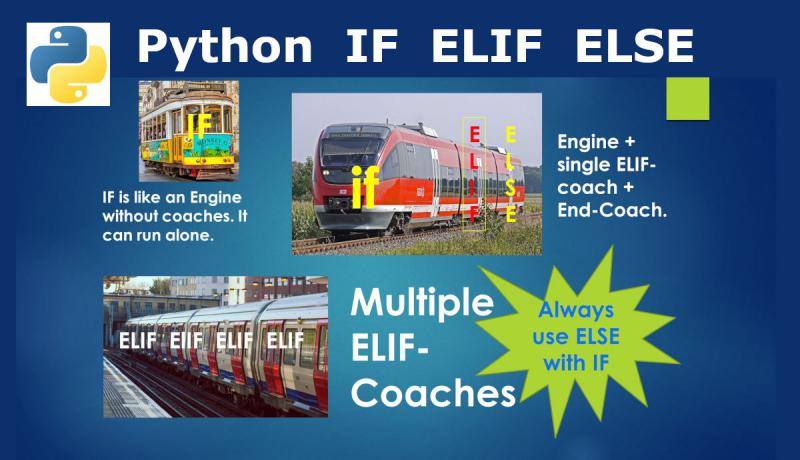
A program statement that can alter the flow of execution is called a Control Statement. Let us know more about Python IF, ELIF and ELSE control statements with examples using this Last Minute Python tutorial.
A condition is an expression that gives the output as a Boolean data-type value like True or False. In Python, any non-zero value is treated as True and a zero is treated as False.
Note: A condition may be simple or complex with any number of operators.
Python IF ELIF ELSE Control Statements
Python offers three keywords namely if, elif and else to alter/control the program flow.
Python strictly depends on indentation which is nothing but the spaces/tabs before each statement to form blocks. The statements under a single control-statement have the same number of spaces/tabs.
Each control statement is explained below.
1. Python IF Control Statement
An IF control statement checks for a condition. If the condition is True, the statements under the IF block will be executed.
You can optionally surround the condition using Parentheses (). In languages like C and Java, Parentheses are mandatory.
The IF statement can be used alone. Note that there is a COLON symbol after the condition indicating the start of the statements-block.
Syntax:
if condition: #statement1 #statement2 #end of if block
Example:
age=10; if age>=6: #if age is more than or equal to 6 print('School Admission Ok') #output #School Admission Ok
2. Python ELSE Control Statement
A Python ELSE-statement is used only in conjunction with an IF statement.
You can use the ELSE statement along with single or multiple ELIF-statements. So, an ELSE statement can come immediately after an IF or an ELIF statement.
The ELSE statement can not be used alone. Note that there should be a COLON after the ELSE keyword.
Syntax:
if condition: #statement1 #statement2 else: #statement3 #statement4 #end of if block
Example:
fuel=15 if fuel>20: print('High Fuel'); else: print('Check Fuel'); #output #Check Fuel
3. Python ELIF Control Statement
The ELIF statements in Python are used to check multiple conditions and execute the corresponding statements. ELIF statements are useful especially when checking ranges.
A single-ELIF or multiple-ELIF statements can appear immediately after an IF statement or before a final ELSE statement.
Python does not allow writing an ELIF statement without an IF statement. The final ELSE statement is optional.
Syntax:
if condition_1: #statement1 #statement2 elif condition_2: #statement3 #statement4 else #statement5 #end of if-elif-else
Example: Below program shows how to use IF-ELIF-ELSE statements to check the range of values and print the Student's grade.
marks=75; if marks>=90: print("Super Score"); elif marks>=80 and marks<90: print("Good Score"); elif marks>=70 and marks<80: print("Medium Score"); elif marks>=60: print("Pass Score"); else: print("Failed"); #output #Medium Score
Python Nested IF ELIF ELSE statements
Nesting of control statements is possible in Python. Nesting is nothing but placing one set of control statements inside other control statements.
Maintaining proper indentation is mandatory with each IF or ELSE or ELIF group.
You can nest an IF statement inside another IF or ELSE statement.
You can nest an IF-ELSE inside another IF or ELSE or ELIF.
You can nest an IF-ELIF-ELSE inside another IF or ELSE or ELIF.
Examples: Below program tries to decide a colour category using nested if-else-elif statements.
r=200; g=150; b=250; if r>100: if g>100: if b>200: print('Good Colour'); elif g>100: if r>200: if b>240: print('Bright Colour'); else: if g>100: if b>200: print('Average Colour'); #output #Good Colour
There are other control statements like Loops in Python. You will learn these in the coming chapters.
You can easily install, compile and run Python programs using our step by step tutorials.
Share this Last Minute Python tutorial on IF, ELIF and ELSE Control Statements with your friends and colleagues to encourage authors.