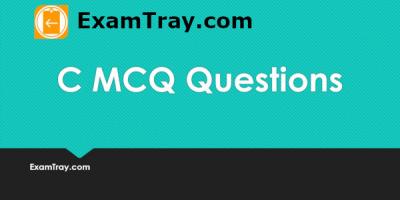
Learn C Programming MCQ Questions and Answers on Loops like While Loop, For Loop and Do While Loop. Loops execute a series of statements until a condition is met or satisfied. Easily attend exams after reading these Multiple Choice Questions.
Go through C Theory Notes on Loops before studying questions.
int main() { int a=14; while(a<20) { ++a; if(a>=16 && a<=18) { continue; } printf("%d ", a); } return 0; }
Between 16 - 18, continue statement skips all other statements below it. So a will not be printed during that time.
15 printed
16 not printed
17 not printed
18 not printed
19 printed
20 printed
for(; ;);
We are not specifying condition to exit the loop. Eg. for(a=0;a<10;a++)
int main() { int a=10, b, c; b=a++; c=++a; printf("%d %d %d", a, b, c); return 0; }
a++ first assigns 10 to b. Next a is incremented separately. ++a increments from 11 to 12. Final ++a value is assigned to the left side variable C.
int main() { int a=0, b=0; while(++a < 4) printf("%d ", a); while(b++ < 4) printf("%d ", b); return 0; }
(++a < 4) first increments and compares afterwards. (b++ < 4) first compares and increments afterwards.
int main() { int a=10,b=20; if(a==9 AND b==20) { printf("Hurray.."); } if(a==10 OR b==21) { printf("Theatre"); } return 0; }
There are no keywords like AND / OR in C language. Logical OR is represented with two Pipes ||. Logical AND is represented with two Ampersands &&.
All remaining characters are special characters or symbols in C Language. 0 to 47, 58 to 64, 91 to 96, 123 to 127.
There were only 128 Characters with 7 Bits in Original ASCII specification. Present character standard in all modern programming languages is UNICODE which covers all languages, Emojis and other special symbols all over the world.