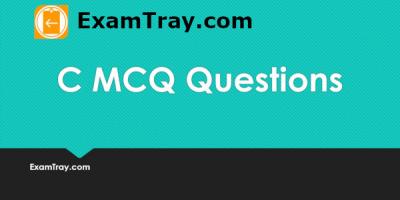
Learn C Programming MCQ Questions and Answers on Switch Case Syntax. Switch case takes only integers. Each Case can check for only Equals To operation. Easily attend exams after reading these Multiple Choice Questions.
Go through C Theory Notes on Switch Case before studying questions.
int main() { int a=5; switch(a) { case 0: printf("0 "); case 3: printf("3 "); case 5: printf("5 "); default: printf("RABBIT "); } a=10; switch(a) { case 0: printf("0 "); case 3: printf("3 "); case 5: printf("5 "); default: printf("RABBIT "); break; } return 0; }
Absence of break; after case statement causes control to go to next case automatically. So after matching 3 with a==3, program prints 3 and control falls down the ladder without checking case again printing everything below it. So Switch checks only once and falls down.
int main() { int a=3; switch(a) { case 2: printf("ZERO "); break; case default: printf("RABBIT "); } }
Notice that it is "default" not "case default".
int main() { int a=3; switch(a) { } printf("MySwitch"); }
Yes. It is okay to omit CASE: statements inside SWITCH construct or block.
int main() { int a; switch(a) { printf("APACHE "); } printf("HEROHONDA"); }
Notice the missing CASE or DEFAULT statements. Still compiler accepts. But without CASE statement nothing will be printed inside of SWITCH.
switch(a) { printf("APACHE "); }
int main() { int a; switch(a); { printf("DEER "); } printf("LION"); }
Notice a semicolon at the end of switch(a);. So, printf DEER is out of SWITCH.
switch(a) { ; } { printf("DEER "); }
int main() { static int a=5; switch(a) { case 0: printf("ZERO ");break; case 5: printf("FIVE ");break; case 10: printf("DEER "); } printf("LION"); }
After matching 5, FIVE will be printed. BREAK causes control to exit SWITCH immediately. Also, using a STATIC variable is also allowed.
int main() { char code='K'; switch(code) { case 'A': printf("ANT ");break; case 'K': printf("KING "); break; default: printf("NOKING"); } printf("PALACE"); }
Any character is replaced by ASCII code or number by the compiler. So it is allowed to use CHARACTER constants for CASE constants.
int main() { char code='K'; switch(code) { case "A": printf("ANT ");break; case "K": printf("KING "); break; default: printf("NOKING"); } printf("PALACE"); }
You can not use STRING constants with DOUBLE QUOTES as CASE constants. Only expressions leading or constants leading to Integers are allowed.
int main() { char code='A'; switch(code) { case 64+1: printf("ANT ");break; case 8*8+4: printf("KING "); break; default: printf("NOKING"); } printf("PALACE"); }
ASCII value of 'A' is 65. So (64+1) matches A. Also expression leading to Integers can be used as CASE Constants.
int main() { char code=64; switch(code) { case 64: printf("SHIP ");break; case 8*8: printf("BOAT "); break; default: printf("PETROL"); } printf("CHILLY"); }
You can not use DUPLICATE SWITCH Case Constants. 64 == (8*).
int main() { int k=64; switch(k) { case k<64: printf("SHIP ");break; case k>=64: printf("BOAT "); break; default: printf("PETROL"); } printf("CHILLY"); }
You can not use comparison operations with CASE statements in SWITCH.
int main() { int k=8; switch(k) { case 1==8: printf("ROSE ");break; case 1 && 2: printf("JASMINE "); break; default: printf("FLOWER "); } printf("GARDEN"); }
(1==8) is false i.e 0. (1&&2) is true AND true i.e true i.e 1. So case allowed first ZERO 0 and then ONE 1. All integer constants are allowed for SWITCH Case Constants.
int main() { int k=25; switch(k) { case 24: printf("ROSE ");break; case 25: printf("JASMINE "); continue; default: printf("FLOWER "); } printf("GARDEN"); }
You can not use CONTINUE; statement as SWITCH is not a LOOP like for, while and do while.
int main() { switch(24.5) { case 24.5: printf("SILVER ");break; case 25.0: printf("GOLD "); break; default: printf("TIN "); } printf("COPPER"); }
You can not use float, double or Strings inside Switch or Switch CASE.