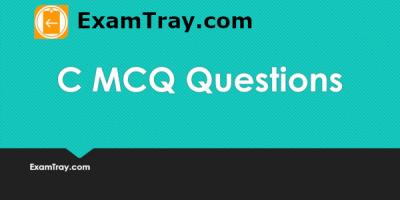
Learn C Programming MCQ Questions and Answers on C Arithmetic Operators like Modulo Division Operator, Plus, Minus, Star and Division Operators. Operator Precedence and Priority is also explained. Easily attend Job interviews after reading these Multiple Choice Questions.
Go through C Theory Notes on Arithmetic Operators before studying questions.
Assignment operator in C has the least priority.
int main() { int a=0; a = 4 + 4/2*5 + 20; printf("%d", a); return 0; }
/ and * has equal priority. But associativity is from L to R.
4 + 2*5 + 20
4 + 10 + 20 = 34
int main() { int a=0; a = 10 + 5 * 2 * 8 / 2 + 4; printf("%d", a); return 0; }
10 + 10*8/2 + 4
10 + 80/2 + 4
10 + 40 + 4 = 54
int main() { int a=0; a = 4 + 5/2*10 + 5; printf("%d", a); return 0; }
5/2 is 2 only because both numerator and denominator are integers. So only int value i.e 2 is the result.
4 + 2 * 10 + 5
4 + 20 + 5 = 29.
int main() { int a=0; a = 10 + 2 * 12 /(3*2) + 5; printf("%d", a); return 0; }
Paranthesis changes the associativity of operators.
10 + 2 * 12 / (3*2) + 5;
10 + 24 / (3*2) + 5;
10+ 24/6 + 5;
10 + 4 + 5 = 19;
int main() { int a=0; a = 10 + 2 * 12 / 3 * 2 + 5; printf("%d", a); return 0; }
10 + 2 * 12 / 3 * 2 + 5;
10 + 24/3*2 + 5;
10 + 8*2 + 5;
10 + 16 + 5 = 31;
int main() { float a=10.0; a = a % 3; printf("%f", a); return 0; }
You can use the operator Modulus Division % with only integers.
error: invalid operands to binary % (have ‘float’ and ‘int’) a = a % 3; ^
int main() { float a=10.0; a = (int)a % 3; printf("%f", a); return 0; }
Type casting from float to int is done by (int).
(int)10.000000 = 10;
10%3 = 1. Reminder of the division of 10 by 3.
%f in printf prints it as 1.000000.
int main() { int a=0; a = 14%-5 - 2; printf("%d", a); return 0; }
14%5 = 4 ( Reminder)
14 % -5 = 4. Yes sign of the reminder is the sign of Numerator.
4- 2 = 2;
int main() { int a= 3 + 5/2; printf("%d", a); return 0; }
Assignment Operator = in C language has the least priority. So the right hand side expression is evaluated first and then assigned to the left side variable.
a = 3 + 5/2;
a = 3 + 2;
a = 5;