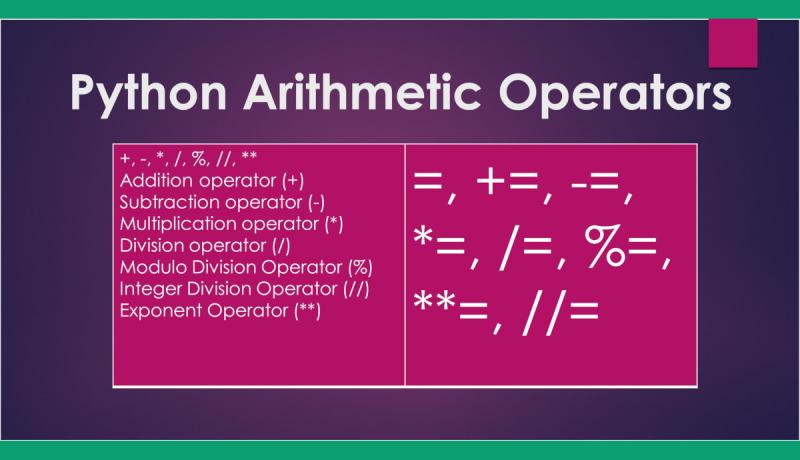
The operators used for doing arithmetic operations are called Arithmetic operators. Addition operator, subtraction operator, multiplication operator, division operator and modulo-division operator are mostly used. Let us know how to use these Python arithmetic operators with examples in this Last Minute Tutorial.
Arithmetic operators work with numeric data types like int, float and complex.
Note: Python programming language does not support increment operator (++) and decrement operator (--).
Python Arithmetic Operators
Operators operate or work on operands.
Python supports the below seven arithmetic operators.
- Addition operator (+)
- Subtraction operator (-)
- Multiplication operator (*)
- Division operator (/)
- Modulo Division Operator (%)
- Integer Division Operator (//)
- Exponent Operator (**)
The modulo division operator gives the remainder of the division operation.
The Integer Division operator or Floor Division operator gives the output of division by removing fractional part or decimal points. So, this Floor division operator does type casting the result to int type.
Exponent operator (**) is also called Power operator. In other programming languages, the caret operator (^) is used for this purpose.
Examples:
a = 10; b = 4; print(a,b) 10 4 print(a+b) 14 print(a-b) 6 print(a*b) 40 print(a/b) 2.5 print(a/a) 1.0 #Division operation always yields float output print(a%b) 2 print(a//b) 2 print(a**b) 10000
Python Arithmetic Assignment Operators or Augmented Assignment Operators
There are 8 arithmetic assignment operators in Python. Out of these, there are 7 augmented assignment operators.
The 'Equals to" operator is represented by "=". This is also called an assignment operator.
Below is the list of Augmented assignment operators. Assume that a and b are two variables or operands.
Operator | Expression | Usage |
Addition Assignment operator (+=) | a = a +b | a+=b |
Subtraction Assignment operator (-=) | a = a - b | a-=b |
Muliplication Assignment operator (*=) | a = a * b | a*=b |
Division Assignment operator (/=) | a = a / b | a/=b |
Modulus Assignment operator (%=) | a = a % b | a%=b |
Floor Division Assignment operator (//=) | a = a // b | a//=b |
Exponent Assingment operator (**=) | a = a ** b | a**=b |
Examples:
a=12; b=5; a+=b print(a) 17 a-=b print(a) 12 a*=b print(a) 60 a/=b print(a) 12.0 a//=b print(a) 2.0 a**=b print(a) 32.0 a = int(a) a%=b print(a) 2
Python Arithmetic Operator Priority
The operator priority or precedence is important to evaluate arithmetic expression involving many operators. The order of evaluation of arithmetic operators is from left to right.
Out of all arithmetic operators, the exponent operator (**) has the highest priority. Addition and Subtraction operators have the least priority. Multiplication, Division, Modulo Division and Floor Division operators are in the equal priority group.
All assignment operators have the least priority. Except "=" operator, all other assignment operators do arithmetic operations only.
Operator | Priority |
Exponent Operator (**) | 1 |
Multiplication (*), Division (/),Modulo Division (%), Floor Division (//) | 2 |
Addition (+), Subtraction (-) | 3 |
=, +=, -=, *=, /=, %=, //= and **= | 4 |
Example: In the below example, * and / have equal priority. So STAR operator on the left is evaluated first.
Arithmetic Expression: 2*3/3+5//2-1 Step 1: 6/3+5//2-1 #first multiplication Step 2: 2.0+5//2-1 #division Step 3: 2.0+2-1 #modulo division Step 4: 4.0-1 #addition Step 5: 3.0 #subtraction
This is it. Practice more examples like these to get a good understanding.
Share this Last Minute Python tutorial on Arithmetic Operators and their Priority with your friends and colleagues to encourage authors.