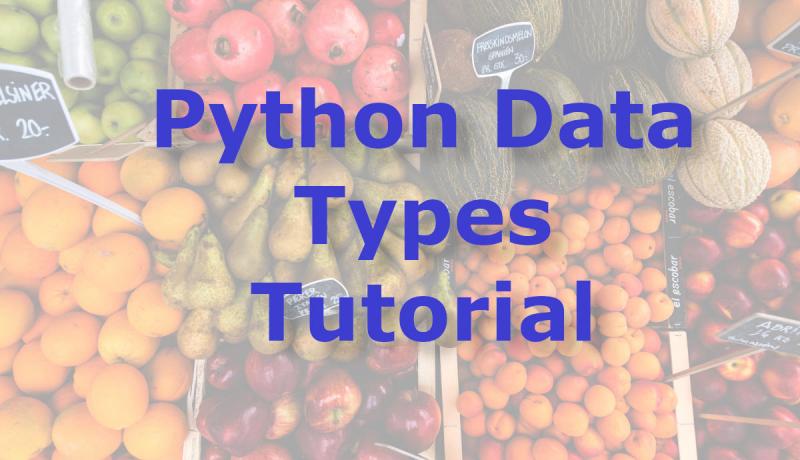
Python is a dynamically Typed language meaning that the type of a variable is determined at runtime based on the type of data assigned to it. You do not need to tell the compiler anything about the type of data or variable at compile time. Let us know more about Python Data types in this last-minute python tutorial.
Note: Every variable is treated as an object in Python. So, it is uncommon to say Primitive and Non-Primitive (Object) types in Python. So, you should refer to these as built-in data types.
Python Sequences is a very big topic. So, a detailed explanation will be given in subsequent chapters.
Python Data Types
The data type is nothing but the Type of the Data. Python has 8 basic data types. There are some more types too.
- Numeric Type (int, float, complex)
- Boolean Type
- String or Text Sequence Type
- Binary Sequence Type (bytes, bytearray, memoryview)
- Sequence Type (list, tuple, range)
- Set Type (set, frozenset)
- Mapping Type (dict)
- None type
1. Numeric Data Type
The numeric data types include int, float and complex types. These are simply numbers in various formats.
1.1 int data type
All integers or whole numbers are treated as int data type values. If an integer is assigned to a variable, the variable is called int variable. The keyword "int" exists.
Syntax examples:
a = 10 b = -20 c = a+b;
1.2 float data type
Any number with a decimal point is treated as a floating-point number or data type. To represent exponents 'e' or 'E' are used. The keyword "float" exists.
Syntax examples:
price = 10.5098 rate = 20.001 percentage = -30.1 exp = 5.5e3 #5.5*10*10*10
1.3 complex data type
The complex data type is used to work with real-world mathematical expressions involving numbers with real-part and imaginary-part. A suffix 'j' or 'J' is added next to the imaginary part.
The keyword "complex" exists.
Syntax examples:
a = 10 + 20j //10 is real. 20j is imaginary. b = 20 + 10J c = a + b print(c) #output #30+30j
2. Boolean Type
A boolean or bool data type has only two values namely "True" and "False". Remember that the first letter is capitalized.
True is treated as an integer 1. False is treated as integer 0.
The keyword "bool" exists.
Syntax Examples:
win = False win2 = 5!=0 #win2 = True c = True a = True + False print(a) #1+0 = 1 b = True and False #False e = True or False #True
3. String or str or Text Sequence Type
A Python string is a sequence of characters. You can combine numbers, special symbols and punctuations with characters.
The keyword used with string operations is "str". Of course, you do not use this keyword every time.
You should enclose a string within a pair of Single-Quotes or Double-Quotes. A multi-line string should be enclosed within a pair of Triple-Single-Quotes or Triple-Double-Quotes.
The index of the first element in a string is 0. The end-index is N-1 where N is the length of the string.
Syntax examples:
str1 = "Hello Python.." print(str1[0]) #prints H str2 = 'Hello CPython.." print(str2[6]) #prints C str3 = '''The quick brown fox jumped over the lazy dog''' str4 = """Jack and Jill went up the hill to fetch water"""
The Strings topic will be discussed in detail in the coming chapters.
4. Binary Sequence Type
Binary sequence data types work with byte-sized integers. The allowed values are from 0 to 255. Only positive numbers are allowed.
4.1 bytes
The "bytes" data type accommodates a group of byte-sized numbers. The individual values can not be modified after creation. Thus it is immutable.
Syntax example:
ary = [1, 5, 6, 2, 7] b1 = bytes(ary) print(b1[0]);
4.2 bytearray
The "bytearray" data type accommodates a group of byte-sized numbers. The individual values can be modified even after creation. Thus it is mutable.
Syntax example:
ary = [10, 8, 6, 3, 4] b2 = bytearray(ary) print(b2[0]);
4.3 memoryview
The "memoryview" data type accommodates a group of byte-sized numbers. The individual values can be modified even after creation. The memoryview expects bytes or bytearray as input.
Syntax example:
ary = [22, 11, 33, 55] bb = bytes(ary) mv = memoryview(bb) print(mv[0]);
5. Sequences
Sequences are nothing but a group of elements like arrays. Sequences allow you to refer to a large number of elements using a single variable name and a subscript. A subscript is surrounded by opening and closing square brackets.
You can put elements of different data types inside a sequence.
Important Sequences are as below.
- List
- Tuple
- Range
5.1 List
The List in Python is mutable, meaning that the individual elements can be modified at any time.
To define a list, you should surround the comma-separated elements by Square brackets.
Syntax Example:
marks = [10, 12, 9, 15] marks2 = list([89, 90, 92, 95]) marks[0] = 22
5.2 Tuple
The Tuple in Python is immutable, meaning that the individual elements can not be modified after creation.
To define a tuple, you should surround the comma-separated elements by Parentheses.
Syntax Example:
rainfall= (10, 12, 9, 15) marks = tuple((89, 90, 92, 95)) marks[0] = 22 #error. can not modify #TypeError: 'tuple' object does not support item assignment
5.3 Range
The Range in Python is immutable, meaning that the individual elements can not be modified after creation.
Syntax Example:
range(stop) #stop is exclusive range(start, stop) #start is inclusive range(start, stop, step) obj = range(5) #numbers from 0 to 4 obj2 = range(5, 10) #numbers from 5 to 9 obj3 = range(11, 20, 2) #numbers from 11. 11, 13, 15, 17, 19. Stepcount=2 list_1 = list(obj) list_2 = list(obj2) tuple_1 = tuple(obj3)
6. Set Data Type
A Set is nothing but a group of unique or distinct elements. The elements inside a set are unordered. So, the elements may not appear in the order of entry.
You should enclose the set elements within Curly Braces (simply braces).
There are two types of sets.
- set
- frozenset
6.1 set data type
The "set" data type allows modifying the individual elements after its creation.
Syntax examples:
rollnum = {3, 6, 8, 2} list_a = [1, 4, 7, 9, 10] #list set_a = set(list_a) #list to set set_b = set('chicken')
6.2 frozenset data type
The "frozenset" data type does not allow modifying the individual elements after its creation.
Syntax examples:
tokens = {3, 6, 8, 2} frozen_set = frozenset(tokens); list_c = [1, 9, 7, 9, 10] #list frozenset_10 = frozenset(list_c) #list to frozenset frozenset_d = frozenset('book')
7. Mapping or dict data type
A Dictionary is a collection of comma-separated Key-Value pairs. Each key and value are separated using a Colon(:) symbol.
Python supports a dictionary type mapping object. The keyword used to define a dictionary object is "dict".
Note: A dict type object expects its keys to be of an immutable type.
Syntax examples:
dict_1 = {5:'honey', 6:'bee', 10:'jar'} print(dict_1) {5: 'honey', 6: 'bee', 10: 'jar'} print(dict_1.keys()) dict_keys([5, 6, 10]) print(dict_1.values()) dict_values(['honey', 'bee', 'jar'])
8. None data type
The None data type in Python is comparable to null data type in Java. None is the default return type of a function or method if you do not write any return statement.
Syntax example 1:
def show(): print('no return') a = show(); print(a); #output no return None
The None data type is also used in default arguments of functions or methods in Python.
Syntax example 2:
def show(a, b=None): if b == None: print(a); else: print(a,b) show('hello'); show('hello','python') #output hello hello python
Python allows you to create custom classes which are treated like custom data types. We shall discuss about functions and classes in subsequent chapters.
Share this Last Minute Python tutorial on Data Types with your friends and colleagues to encourage authors.