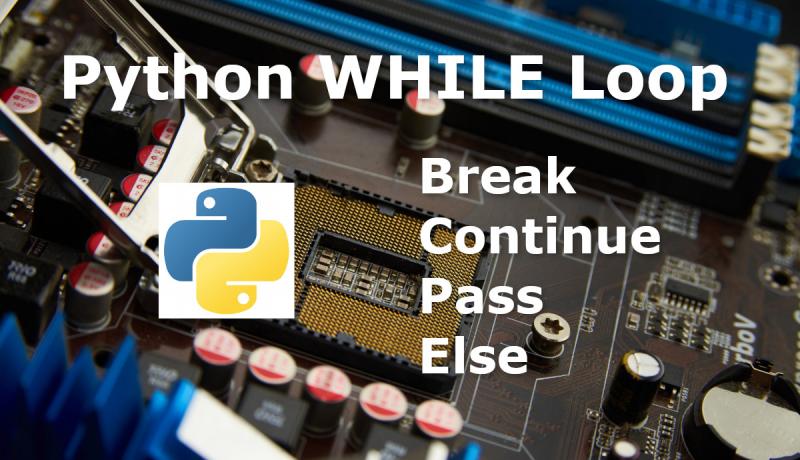
Python language supports loops or iterations. A program block that repeatedly executes a group of statements based on a condition is called a Loop. Let us know more about a Python WHILE loop with a break, continue and pass control statements with examples.
Note: Main Keywords used in this tutorial are while, break, continue, pass and else.
Unlike in languages like C and Java, Python supports only two loops.
- WHILE loop
- FOR Loop
The Python-While loop works with a separate loop-counter. The FOR loop works only with a group of elements like List, Tuple, Range, Array etc.
Python WHILE Loop
Python While loop is a control statement that accepts a condition as the input. As long the condition is True, loop-block statements are executed for each iteration of loop-counter. If you forget to increment or decrement the counter, you will end up with an infinite loop.
Any infinite loop consumes more RAM (Random Access Memory) and crashes the PC.
The condition of a WHILE loop should yield a Boolean value like True or False. The condition may contain a number of sub-conditions separated by Boolean operators.
Syntax:
while condition: statement1; statement2; #end of while
Example:
#program to print numbers 1-10 c=1; #loop counter while c<=10: print(c, end=' '); c = c+1; #incrementing counter #end of while #output #1 2 3 4 5 6 7 8 9 10
You can use the Python WHILE loop with three statements namely Break, Continue and Pass. You can combine all or any one of these special statements with the While loop.
1. Python WHILE with BREAK
Some times, it is necessary to come out of a loop based on certain conditions. Hence a BREAK statement is used in Python to break the While loop (or even FOR loop or any loop).
Once the Python runtime encounters this BREAK statement, the control goes to the first statement after the While-loop. All the statements below the break statement and within the While-block are not executed (ignored). A Python Break statement is usually kept inside an IF or ELSE block.
Syntax:
while condition: statement1; break statement2; #end of while
Example: The below program prints Even numbers up to 20 using a WHILE and BREAK control statements.
#program to print even number upto 20 #This program uses BREAK statement c=1; while True: if c%2==0: print(c, end=' '); c = c+1; if c>20: break; #end of while #output #2 4 6 8 10 12 14 16 18 20
2. Python WHILE with CONTINUE
The Python CONTINUE statement is the reverse of the BREAK statement. When the Python runtime encounters this CONTINUE statement, the control goes back to the beginning of the loop. So, you should increment or decrement the loop-counter before writing any CONTINUE statement to avoid infinite loops.
Remember that the Continue statement does not stop or halt the loop. It just instructs to continue with the next iteration.
Syntax:
while condition: statement1; continue; statement2 #end of while
Example:
#program to print odd numbers upto 20 till=0; while till<20: till = till+1; if(till%2 == 0): continue; print(till, end=' '); #end while #output 1 3 5 7 9 11 13 15 17 19
3. Python WHILE with PASS
Along with the Break and Continue statements, Python another control statement that does really nothing. The PASS statement is a dummy statement that tells the Runtime to simply ignore it and continue executing the next line of code.
Syntax:
while condition: statement1; pass; statement2; #end of while
Example:
#program to print numbers divisible by 3 count=0; while count<=10: count+=1; if count%3 ==0: print(count, end=' '); else: pass; #end of while #output #3 6 9
4. Python WHILE with ELSE Clause
Python Else-Clause in combination with a WHILE-loop is executed whether the loop condition is True or False. The ELSE clause usually contains the code for clean-up. You can use the data processed within the WHILE loop inside the ELSE clause. You can a process list of items for example.
This else clause is different from the IF-ELSE control statements where the ELSE part is not executed if the IF part works.
#program with WHILE and ELSE numbers=[2,5,7,15,9]; i=0; while i<5: print(numbers[i]); i+=1; else: print('\nWHILE ends. Always Executed'); #end of else. end of WHILE
This is about a Python WHILE loop. Practice more examples like the above for better understanding Python language.
In the coming chapters, you will learn about Python FOR loop with Break, Continue and Pass.
Share this Last Minute Python tutorial on WHILE Loop with Break, Continue, Pass and ELSE Control Statements with your friends and colleagues to encourage authors.