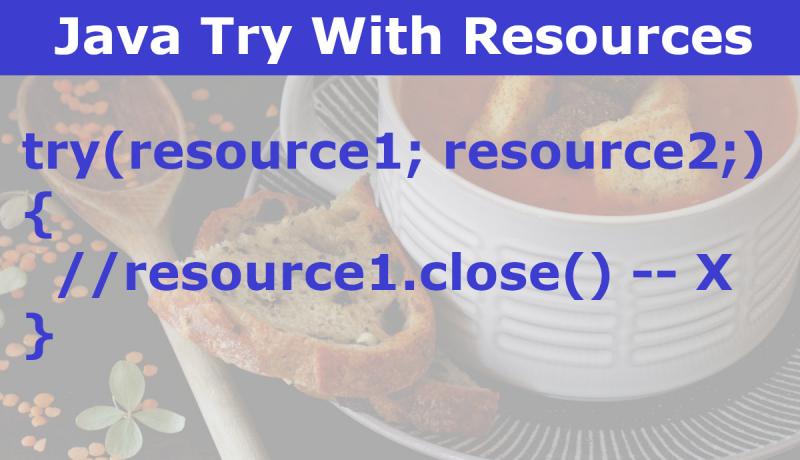
A Java 7 try-with-resources block automatically closes any opened resources even if you do not close. It is like the regular try block with the extra support of Parentheses "( )" to initialize multiple Resources. The keyword used is still "try" only but not "with" or "resources". Let us learn more in this Last Minute Java tutorial with examples.
Know the basics of Java Exception Handling before reading this tutorial.
Java Try-With-Resources or Try With Resources explained
The problem with regular try-catch-finally blocks is that the resources opened by you should be closed by you explicitly to free the locked resource and the memory. When an exception occurs, the program's normal flow is interrupted. Writing duplicate code blocks for releasing the opened resources for all possible scenarios is not suggested. So, Java 7 came up with the idea of a try-with-resources block feature.
Syntax:
try(RESOURCE_1; RESOURCE_2;) { //CODE //Closing RESOURCE 1 or 2 is not required. }
Objects of any class that implements java.lang.AutoClosesable or java.io.Closeable can be used inside a try-with-resources block. You can initialize different objects in multiple-lines or a single-line separated by semicolons (;). This block follows the usual Java syntax for writing multiple statements in Java. There is nothing new.
Let us see how to close a File Resource without using a try-with-resources in the below program. We initialized a BufferedWriter object inside TRY as a resource.
import java.io.BufferedWriter; import java.io.FileWriter; public class TryWithoutResourceExample1 { public static void main(String[] args) { BufferedWriter bw = null; try { bw= new BufferedWriter(new FileWriter("def.txt",true)); bw.append("Appending text"); bw.newLine(); bw.flush(); bw.close(); System.out.println("Successfully opened the file DEF.txt and written.."); } catch(Exception e) { e.printStackTrace(); } finally { try { bw.flush(); bw.close(); System.out.println("Closed inside FINALLY.."); } catch(Exception e3) { e3.printStackTrace(); } } //end of finally } //end of main } //end of class //OUTPUT Successfully opened the file DEF.txt and written.. java.io.IOException: Stream closed at java.base/java.io.BufferedWriter.ensureOpen(Unknown Source) at java.base/java.io.BufferedWriter.flushBuffer(Unknown Source) at java.base/java.io.BufferedWriter.flush(Unknown Source) at TryWithoutResourceExample1.main(TryWithoutResourceExample1.java:30) //DEF.txt contents after 2 writes Appending text Appending text ...
In the above example, we have kept the code to close a file resource in a FINALLY block. There also we have used a nested try-catch inside. The reason for putting the code inside a FINALLY block is that it will be executed even if other exceptions happen.
We can avoid the Stream Closed IOException in the above program, using an IF-ELSE statement inside the finally-block. Just assign a null value to the variable "bw" after the bw.close() statement. In the finally-block, if bw is already null, do not try to close the file resource again.
Let us minimise the above Java code using a try-with-resources block as in the below program. We try to write some data to a file abc.txt.
import java.io.BufferedWriter; import java.io.FileWriter; import java.util.Calendar; public class TryWithResourceExample1 { public static void main(String[] args) { try(BufferedWriter bw = new BufferedWriter(new FileWriter("abc.txt"));) { Calendar cal = Calendar.getInstance(); bw.write("Current Time = " + cal.get(Calendar.HOUR_OF_DAY) + ":" + cal.get(Calendar.MINUTE)); System.out.println("Successfully Written to a file.."); //bw.flush(); //bw.close(); //bw will be closed by try automatically } catch(Exception e) { e.printStackTrace(); } } } //OUTPUT Successfully Written to a file..
Observe that we have not closed the file resource BufferedWriter (bw) inside a try or catch block. We have not even used finally block to close such resources. We saved so much code. This is the advantage of using a Java try-with-resources block.
Note: The object references used in the Java Try With Resources block can not be re-initialized as these variables are treated like FINAL Variables by default.
List of Classes implementing java.lang.AutoCloseable
Here is the list of some well know classes implementing java.lang.AutoCloseable.
- FileReader
- FileWriter
- BufferedReader
- BufferedWriter
- FileInputStream
- FileOutputStream
- BufferedInputStream
- BufferedOutputStream
- Scanner
- StringReader
- StringWriter
- InputStream
- OutputStream
- PipedReader
- PipedWriter
Share this Last Minute Java TRY WITH RESOURCES tutorial with your friends and colleagues.