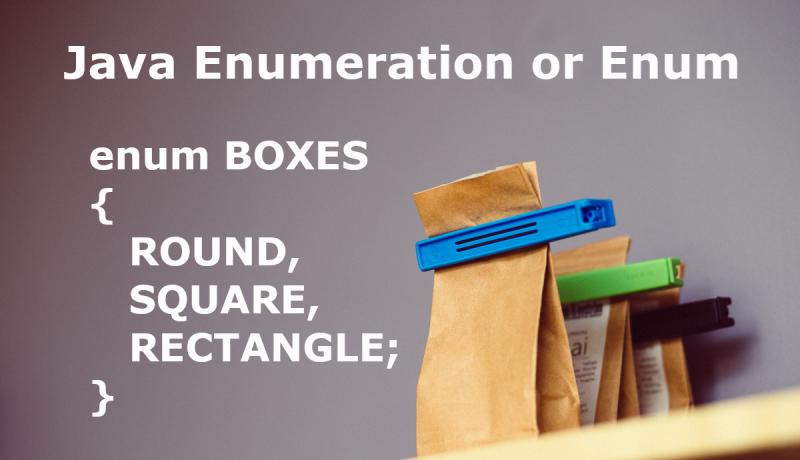
Java Enumeration or Enum is nothing but a Class with a List of Constants, Constructors, Instance Variables and Instance Methods. Enum is like a custom data type. The enumeration constants have an index or ordinal which starts with ZERO just like an array. Let us know more in this Last Minute Java Tutorial.
Note: Java 1.5 introduced this new feature Enumeration to easily manage constants.
Java Enumeration or Enum Explained
Enumeration or enum is designed for the purposes of defining variables that can accept only a predefined set of constants as values. Each enum constant or variable can use inherited methods like name, ordinal, equals, compareTo, values and valueOf.
These constants are used to indicate status or progress in many Java applications. Examples of Enum in real-world are ASSIGNED, PENDING, IN_PROGRESS, CLOSE, RED, GREEN, WAITING etc. These are easy to understand and use.
Enumeration constants are implemented like objects. Java Enumeration can be implemented in two ways.
- Enum with a set of constants
- A Class like Enum implementation with dedicated constructors, instance variables and methods along with Constants. Each constant has a separate copy of these variables, methods and constructors.
Enum (Enumeration) Syntax 1:
enum NAME {CONSTANT1, CONSTANT2.. ;}
In the above enumeration syntax, the semicolon at the end of the list of Constants is mandatory only if the enum contains variables and methods.
Enum (Enumeration) Syntax 2:
enum NAME { CONSTANT1, CONSTANT2..; data_type VARIABLE1; data_type VARIABLE2; NAME(PARAM) //constructor with parameters { //statements } return_type METHOD1(PARAMS) { //statements } //.. }
The second syntax of Enum defines constructors, variables and methods too similar to a regular class. Java enumeration also supports overloading of constructors and overloading of methods. The main difference between an enum and a class is that enumeration variables or objects will be created without using the "new" keyword.
Enums can be defined in two places.
- Outside a class or interface
- Inside a class or interface but outside of any method
Rules to Define Names or Identifiers for Enum and Enum-Constants
Below are the rules to define an Enumeration with constants.
- The name of an Enum or Enum-constant can not be the name of a keyword.
- The names of Enum and Enum-constants can only start with a Letter, Dollar ($) or Underscore (_).
- The names of Enum and Enum-constants can contain numbers.
- You can not declare numbers as enum constants. A number is not a valid identifier.
Methods of an Enumeration or Enum
All Enumerations or enums implicitly inherit or extend from a superclass "Enum". The Enumeration class java.lang.Enum provides a list of useful methods that can be used by all enum variables or constants.
- name() - gets the string name of an enum constant.
- ordinal() - gets index or position of an enum constant.
- equals(Object) - compares two enum variables and returns a boolean value.
- compareTo(enum-type) - compare two enum variables or constants and returns an int value.
- values() - returns an array of all enum constants. Using this array, you can use a loop to iterate through all the elements
- valueOf(String) - returns an enum constant corresponding to the String-name passed.
Example Program 1: Looping through Enum constants
We have used a for-each loop to iterate through all elements of an Enumeration. Elements are nothing but the individual constants. The below program uses all the popular methods of an Enum.
EnumExample1.java
enum FRUITS { MANGO, GRAPE, BERRY, GUAVA; } public class EnumExample1 { public static void main(String[] args) { //declare an enum variable FRUITS f1; //assigning a constant value to enum variable f1 = FRUITS.MANGO; //printing enum-variable System.out.println("f1 = " + f1); System.out.println("Printing all enums.."); //advanced for-loop or for-each loop for(FRUITS item: FRUITS.values()) { System.out.println(item.ordinal() + ". " + item); } //using method name() System.out.println(f1 + " name= " + f1.name()); //using method valueOf() System.out.println(FRUITS.valueOf("BERRY")); //using method equals() System.out.println("f1 == FRUITS.MANGO -->" + (f1.equals(FRUITS.GRAPE))); //using method compareTo() System.out.println("BERRY-MANGO =" + FRUITS.BERRY.compareTo(FRUITS.MANGO)); } } //OUTPUT f1 = MANGO Printing all enums.. 0. MANGO 1. GRAPE 2. BERRY 3. GUAVA MANGO name= MANGO BERRY f1 == FRUITS.MANGO -->false BERRY-MANGO =2
Example Program 2: Using Enumeration or Enum in Switch Statements & Interfaces
Java enumeration constants can be used as Switch-case constants. Remember that the CASE-CONSTANTS do not use the Enum name. The below program shows the usage of switch, constructors, variables and methods. It also demonstrates how to implement an interface using the keyword "implements".
EnumerationExample2.java
interface Interface1 {int getStock();} enum FURNITURE implements Interface1 { CHAIR(5), SOFA(10), TABLE, SHELF(9); int stock; //enum variable FURNITURE(int num) //enum constructor { stock = num; } FURNITURE() //enum default constructor { stock = 0; } public int getStock() //enum method { return stock; } } public class EnumerationExample2 { static void show(FURNITURE fm) { switch(fm) { case CHAIR: System.out.println("CHAIR stock = " + fm.stock); break; case SOFA: System.out.println("SOFA stock = " + fm.stock); break; case TABLE: System.out.println("TABLE stock = " + fm.stock); break; case SHELF: System.out.println("SHELF stock = " + fm.stock); break; default: System.out.println("Unknown Enum constant.."); } public static void main(String[] args) { FURNITURE fnt1 = FURNITURE.CHAIR; FURNITURE fnt2 = FURNITURE.TABLE; show(fnt1); show(fnt2); } } //OUTPUT CHAIR stock = 5 TABLE stock = 0
Note: A Java enumeration can not extend or inherit another Enumeration or Class.
Share this Last Minute Java tutorial on Enumeration with your friends and colleagues to encourage authors.