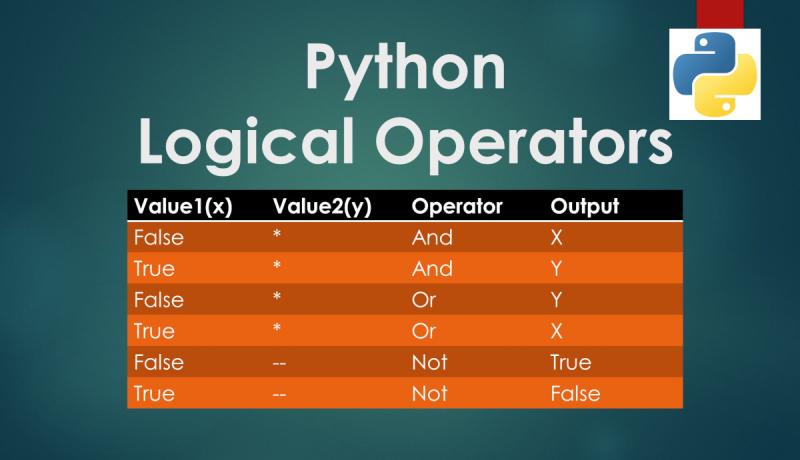
Python language supports Logical operators to form complex conditions. These are different from the logical operators used in C and Java. Let us know more in this Last Minute Python Logical operators and priority tutorial using examples.
Note 1: Python Logical Operators are NOT Symbols but keywords. Also, remember that a logical operation may result in either a Boolean or bool type value or an object.
Note 2: Python Logical operators and Boolean operators are different. In C and Java, boolean and logical operations are one and the same.
Python Logical Operators
Python supports 3 logical operators namely "and", "or" and "not". The first letter of each logical operator's name is not capitalized. These operators allow you to create compound conditions that contain two or more conditions.
These logical operators can be used inside an IF, ELIF and WHILE constructs.
In other words, you can group a number of individual conditions into a single complex condition using these logical operators.
Any non-zero value is considered as True in Python and a zero value is considered as False. So a single condition may contain Arithmetic Operations, Relational Operations or direct Objects.
Python logical operators work with two operands namely left-operand and right-operand in general.
List of Python Logical Operators:
SNO | Logical Operator |
1. | and |
2 | or |
3. | not |
1. AND Logical Operator
If the left operand is False, the output is False. If the left operand is True, the output depends on the right-operand. So, the "and" operator exhibits short-circuit behaviour.
And Truth Table:
Value1(x) | Value2(y) | Output |
False | False | x |
False | True | x |
True | False | y |
True | True | y |
Example:
a=10; b=20; c=0; print(a and b) #output #20 if(a and b): print("LION") #output #LION print(c and a) #output #0 if(c and a): print("CAT") #output #no output
2. OR Logical Operator
If the left operand is True, the output is True. If the left operand is false, the output depends on the right-operand. So, the "or" operator exhibits short-circuit behaviour.
Or Truth Table:
Value1(x) | Value2(y) | Output |
False | False | y |
False | True | y |
True | False | x |
True | True | x |
Example:
x=20; y= 30; z=0; print(x or y) #output #20 print(z or y) #output #30
3. NOT Logical Operator
The Not operator returns the opposite of the input boolean value i.e True for False and False for True.
Not Truth Table:
Value | Output |
True | False |
False | True |
Example:
p=30; k=0; print(not p) #output #False print(not k) #output #True if(not k): print("GOAT") #output #GOAT
Python Logical Operator Priority or Precedence
Python Logical "not" operator has the highest priority among the three operators. The logical "and" operator has the least priority.
If two or more logical operations are present in a compound condition, the order of evaluation is from left to right.
The logical operators' group has a lesser priority than Arithmetic operators, Bitwise operators, Relational operators and Assignment operators.
Python Logical Operator Priority table is given below.
Logical Operator | Priority |
not | 1 |
or | 2 |
and | 3 |
In the coming chapters, you will know how to use Python Boolean operators.
Share this Last Minute Python tutorial on Logical Operators and their Priority with your friends and colleagues to encourage authors.