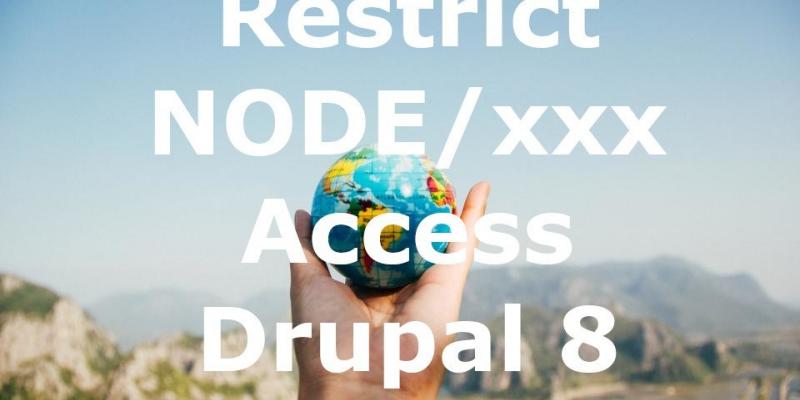
Drupal is a CMS (Content Management System) made up of a number of nodes of different types. Each node represents information of one type. Some times it is necessary to block or ban or restrict access to a node in Drupal 8. Using this Drupal tutorial, you can programmatically block access to node using PHP language.
Block or Ban or Restrict Node Access in Drupal 8
First create new module by name mymodule.module. Inside the module, we make use of a predefined Drupal 8 Function mymodule_node_access to decide whether to allow or block or ban or Restrict access to a node or node type. You can block all node accesses in node/xxx or node/xx format literally. You can even check user roles to allow access to node in Drupal 8. Restriction of access to specific node types increases security of your Drupal site.
mymodule.module
<?php use Drupal\Component\Utility\SafeMarkup; use Drupal\Core\Entity\EntityInterface; use Drupal\Core\Session\AccountInterface; use Drupal\node\NodeInterface; use Drupal\Core\Access\AccessResult; function mymodule_node_access(\Drupal\node\NodeInterface $node, $op, \Drupal\Core\Session\AccountInterface $account) { /*Block access to Nodes in the form **** node/xxx ****/ $path = \Drupal::request()->getPathInfo(); //drupal_set_message($path); $arg = explode('/',$path); $n = 0; try{ if(count($arg)>=3) { $n = $arg[2]; } $n = ($n == (int) $n) ? (int) $n : (float) $n; } catch (Exception $e) { $n = 0; } $roles = \Drupal::currentUser()->getRoles(); if(in_array('admin', $roles)) { return \Drupal\Core\Access\AccessResult::allowed(); } else { if( (count($arg) >= 2) && ($arg[1] == 'node') && ($n >= 0)) { return \Drupal\Core\Access\AccessResult::forbidden(); } else if($node->getType() == 'technology') { //If article type is Technology, then block access blindly return \Drupal\Core\Access\AccessResult::forbidden(); } else { return \Drupal\Core\Access\AccessResult::neutral(); } } } //There is no ending ?> in a .module file
The main question about the need for blocking access to a node is that any anonymous user can go through all nodes of your entire drupal site using http://sitename/node/xx format in any browser if not blocked properly.
http://sitename.com/node/125 will be blocked http://sitename.com/iphone-10-x-specifications will be allowed to open. Both the links point to the same article or node. But the node/xxx format will be affected by Bot activities.
You can also block or allow a node to be viewed based on User Role or Node Type (eg. technology) if required. We allowed admin to access all types of nodes in IF condition by checking $roles. Outside persons can not guess article URLs and directly strip or copy content for misuse.
Though there are some ready made modules to block access to a particular node type based on User Role, this type of fine control over the nodes or node types is not possible with those modules.
You can try Node View Permissions module which is stable and secure as of now. You can set only two states to allow or block each user type or each node type. Usually, it is better to write small PHP code to achieve maximum when compared to these modules.