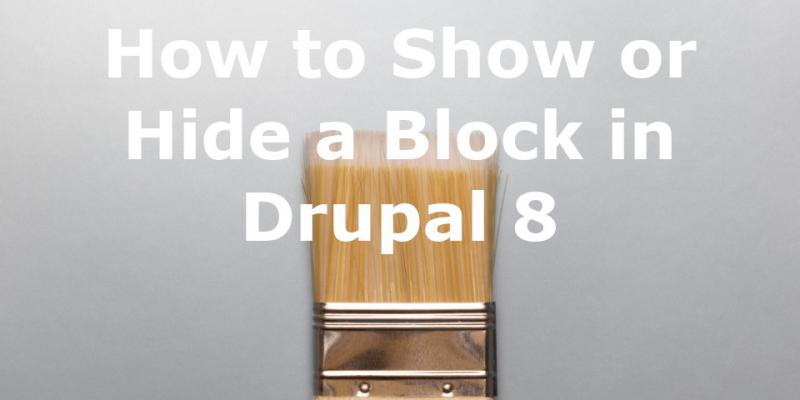
Drupal 8 is made up of a number of Blocks. Each block represents one type of information. Also, each block has a place holder where it will be displayed. Some times it is necessary to show or hide blocks based on User Roles, specific path or pages. Visibility changes the way your Drupal Blocks appear to each user admin or anonymous.
Show or Hide Block in Drupal 8 Programmatically
You need a module to write code for restricting block access. So, create a Drupal 8 Module with name mymodule.module. Inside the module, we are going to use hook_block_access function provided by drupal 8 to do customization. Now, we shall hide a block using PHP programmatically.
How to Find Block Name in Drupal 8
To find a block name, go to Structure and choose Block Layout. You can see a list of blocks from top to bottom. Against each block, there is a Configure Button.
Now click on Configure button. Just check the URL for block name of “Site Branding” for example.
BlockName: mybootstrap_branding
How to restrict or hide a block in drupal 8 to change Visibility
mymodule.module
<?php
use Drupal\block\Entity\Block;
use Drupal\Core\Session\AccountInterface;
use Drupal\node\NodeInterface;
use Drupal\Core\Access\AccessResult;
function mymodule_block_access(Block $block, $operation, AccountInterface $account)
{
if ($operation == 'view')
{
if($block->id() == 'mybootstrap_branding')
{
$roles = \Drupal::currentUser()->getRoles();
//anonymous
//adminstrator
if(in_array('editor', $roles))
{
//Hide the block for specific user roles like editor
return AccessResult::forbiddenIf(true)->addCacheableDependency($block);
}
//Now based on Node Pages, Hide the block
$node = \Drupal::routeMatch()->getParameter('node');
if (($node instanceof \Drupal\node\NodeInterface) && ($node->gettype() != 'technologyarticle') )
{
//Hiding the block if the node is not 'technologyarticle' type
return AccessResult::forbiddenIf(true)->addCacheableDependency($block);
}
}
}
//There is no ending ?> in a .module file
In the code above, we are hiding the block mybootstrap_branding based on Node Type or Content Type or Path or User Role. You can add your own rules to suit your needs to change visibility of a Drupal 8 Block.
You can also restrict a node access in Drupal 8 based on various conditions like User Roles or Path. Drupal 8 has hooks for various purposes. We have to use those correctly.