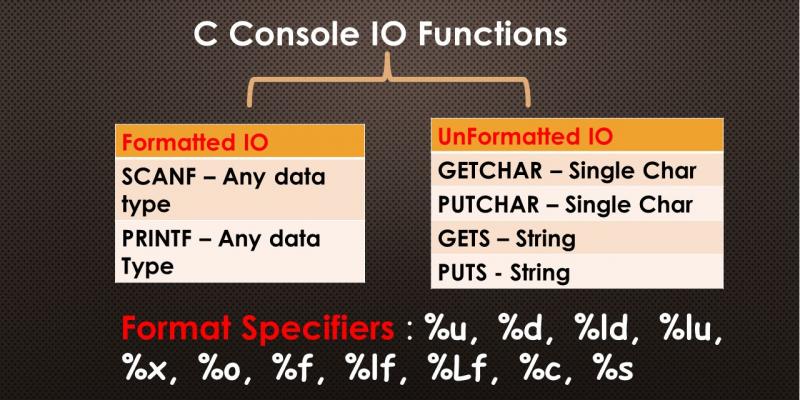
C Programming language provides Console IO functions and Format Specifiers to help programmers Read and Write data.
C Format Specifiers and Console IO Functions
There are two types of Input Output Functions.
- Console IO
- File IO
1. Console IO functions help to read data from Keyboard and Print / Display data to the Monitor.
2. File IO functions help to read data from Files on Disk and Write data to the Disk.
C Format Specifiers are used in IO functions to display data in a specified format so that it can be read easily and printed for reports.
Escape Sequence Characters in C
Escape sequence characters in C language are not processed like normal characters. An Escape sequence starts with a BACK SLASH ( \ ) character. An immediate character next to Back Slash is also treated part of the Escape Sequence. Examples are Escape sequences are New Line Character, Line Feed, Form Feed etc.
SNO | Escape Sequence | Meaning |
---|---|---|
1 | \n | New Line - Cursor goes to next line beginning |
2 | \r | Carriage Return - Cursor goes to same line beginning |
3 | \b | Back Space - One character is deleted backwards |
4 | \t | Horizontal Tab - Cursor moves forward to 4 or 8 spaces |
5 | \f | Form Feed - Makes the printer to go to the beginning of next page |
6 | \a | Audible Alert - Plays a sound |
7 | \' | Single Quote - Prints a single quote |
8 | \" | Double Quote - Prints a double quote |
9 | \\ | Back Slash - Prints a back slash |
Example 1:
int main() { printf("TOM\nCAT"); } //OUTPUT // \n produces new line //TOM //CAT
Format Specifiers in C Language
A Format Specifier specifies type of data to be Read from Keyboard or Printed on Screen. Types of data available are char, short, int, long, float, double and long double.
List of format specifiers is given below.
SNO |
Format Specifier | Data Type |
---|---|---|
1 | %d or %l | short signed |
2 | %u | short unsigned |
3 | %ld | long signed |
4 | %lu | long unsigned |
5 | %x | unsigned hexadecimal |
6 | %o | unsigned octal |
7 | %f | float |
8 | %lf | double |
9 | %Lf | long double |
10 | %c | signed or unsigned char |
11 | %s | string |
You can also specify Field Width and Precision as part of a format specifier. Filed Width (W) represents number of COLUMNS to be reserved. Precision (P) represents Number of Digits after Decimal Point. It is used in the below format. Symbols '+' or '-' or used to specify Right Alignment and Left Alignment of text respectively. Plus sign is applied by default. So there is no need to type or specify + symbol.
Precision is used along with float and double data types.
Note: If less columns are occupied with data, blanks will be shown in place holders. Blanks will appear on right if the text is Left Justified. Blanks will appear on Left if the text is Right Justified.
%(+ or -)(W.P)(Type Specifier) eg. %10d Right Justification %-10d Left Justification %12.2f, %-12.2f %16s, %-16s
Note: You can truncate right side of a String or Char Array using Field Width Specifiers as part of Format Specifiers. You can printf only first N character by using %(W.N)s notation with strings.
Example 1
int main() { printf("123456 123456\n"); printf("%6d %6d\n", 123, 45678); printf("%-6d %-6d", 4891, 78); return 0; } //OUTPUT 123456 123456 123 45678 4891 78
Example 2: Truncating part of String with Field Width Specifiers
Strings or char arrays can be truncated using %(W.P)s format specifier. W is the number of columns to be reserved and P is number of characters to be shown counting from Left.
int main() { printf("123456 123456\n"); printf("%6s %6s\n", "abc", "defgi"); printf("%-6s %-6s\n", "abc", "dghi"); printf("\n123456\n"); //prints only first 2 of 6 characters printf("%6.2s\n", "abcdef"); printf("%-6.3s\n", "abcdef"); return 0; } //OUTPUT 123456 123456 abc defgi abc dghi 123456 ab abc
Example 3: formatting string with float and double
Float and Double numbers can be formatted specifying Precision or Number of Digits after decimal point to be shown. Total number of digits are represented by W and Precision digits are part of W number. So here in this example, %6.2f specifies a total of 6 digits out of which 2 digits will be used to show precision.
int main() { printf("NAME=%s\n, Score=%6.2f", "TOMMY", 97.578963); return 9; } // // 97.57 //double value is type casted to float
//97.5 is double. //double value is type casted to float
Console IO Functions in C Language
Console is a combination of Keyboard and Monitor. Console IO functions are classified in to two types.
- Formatted Input Output Functions
- Unformatted Input Output Functions
1. Formatted Input Output Functions
Formatted IO is done in C using only two functions namely PRINTF and SCANF. Formatted IO functions work with all types of data namely integers, real number and character data.
int main() { char name[10]; int age; printf("Enter a name: "); scanf("%s, name); printf("Enter age: "); scanf("%d", &age); printf("Name=%s, age=%d", name, age); return 9; }
There are functions like SPRINTF and SSCANF aimed at storing the formatted data to a CHAR Array or String. Functionality of a SPRINTF is similar to PRINTF. Functionality of SSCANF is similar to SCANF. In the below example, keyboard data is read into a temporary String str.
int main() { char str[20]; char name[10]; int age; sprintf(str, "Name=%s, age=%d", "JIMMY", 25); printf("%s", str); sscanf(str, "%s%d",name, &age); printf("\n%s", str); }
2. Unformatted Input Output Functions
Unformatted IO is done in C using only Four functions namely GETCHAR, PUTCHAR, GETS and PUTS. Unformatted Input Output functions are meant only to deal with Character type data and String type data. You can not process numbers using these functions.
Note 1: GETCHAR and PUTCHAR functions work with only SINGLE Character.GETS and PUTS functions work with character array or string data.
Note 2: GETS function can read a string of characters with SPACES and TABS. Scanf can not read a line of text with spaces and tabs.
Example 1: Using GETCHAR and PUTCHAR functions:
int main() { char ch; printf("Enter a character: "); //Press enter after typing a character ch = getchar(); //Now print using PUTCHAR printf("\nEntered="); putchar(ch); return 9; }
Example 2: Using GETS and PUTS functions:
int main() { char ch; printf("Enter a character: "); //Press enter after typing a character ch = getchar(); //Now print using PUTCHAR printf("\nEntered="); putchar(ch); return 9; }
C Online Test on Console IO and Format Specifiers
1 | Console IO, Formatted Output and Format Specifiers - Online Test 1 |