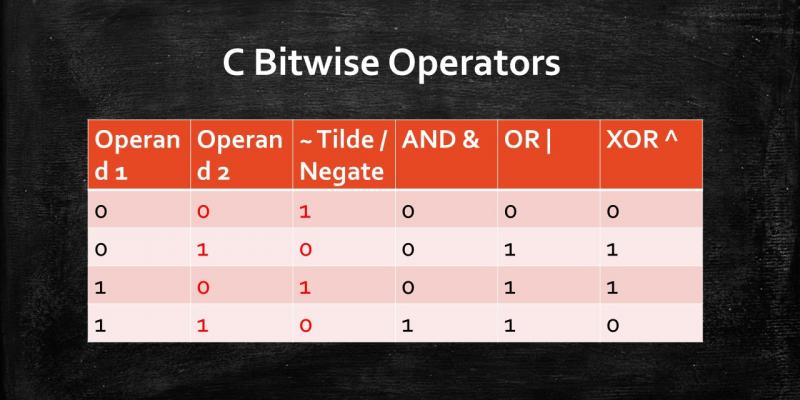
C programming language provides various operators like Arithmetic, Logical, Conditional and Bitwise operators. Bitwise operators deal with Bits of data instead of Bytes of data.
Bitwise Operators in C
There are six Bitwise Operators in C.
- ~ TILDE
- << Left Shift
- >> Right Shift
- & Bitwise AND
- | Bitwise OR
- ^ Bitwise XOR ( Exclusive OR)
1. TILDE ~ Operator
TILDE ~ Operator is also called Bitwise One's Complement Operator. 1's complement operator simply replaces 1s with 0s and 0s with 1s.
Op1 | Result |
---|---|
0 | 1 |
1 | 0 |
//Binary of number 6 = 0110 int a= 6; printf("%d %d", a, ~a); //0110 -> 1001 //OUTPUT: 6 9
2. << Left Shift Operator
Left Shift operator shifts bits of a number from Right to Left. Gaps created on the Right side are filled with Zeroes. Bits on the left side are removed. So, <<N removes N bits on the left side.
//Binary of number 6 = 0110 int a = 6; printf("%d %d", a, a<<2); //Two Zeroes are filled right side. // 0110 -> 1000 //OUTPUT: 6 8
3. >> Right Shift Operator
Right Shift operator shifts bits of a number from Left to Right. Gaps created on the Left side are filled with Zeroes. Bits on the Right side are removed. So, >>N removes N bits on the Right side.
//Binary of number 6 = 0110 int a = 6; printf("%d %d", a, a>>2); //Two Zeroes are filled left side. // 0110 -> 0001 //OUTPUT: 6 1
4. & Bitwise AND Operator
Bitwise AND Operator & multiplies bit by bit. So 1 multiplied by 1 gives 0. Anything Multiplied by 0 gives 0.
Op1 | Op2 | Result |
---|---|---|
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
//Binary of number 6 = 0110 //Binary of number 3 = 0011 int a = 6, b=3; printf("%d", a & b); //0010 //OUTPUT: 2
5. | Bitwise OR Operator
Bitwise OR Operator | gives a result of 1 if at least one input or operand is 1.
Op1 | Op2 | Result |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 1 |
//Binary of number 6 = 0110 //Binary of number 3 = 0011 int a = 6, b=3; printf("%d", a | b); //0111 //OUTPUT: 7
6. ^ Bitwise Exclusive OR
Bitwise XOR Operator or Exclusive OR operator gives an output of 1 if two operands are different. That means, only 0 and 1 combination gives output as ONE.
Op1 | Op2 | Result |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
//Binary of number 6 = 0110 //Binary of number 3 = 0011 int a = 6, b=3; printf("%d", a | b); //0101 //OUTPUT: 5
C Bitwise Operators Examples Table
NO | Operator | Expression | Result |
---|---|---|---|
1 | ~ | ~1010 | 0101 |
2 | & | 1010 & 0011 | 0010 |
3 | | | 1010 | 0011 | 1111 |
4 | << | 1010 << 2 | 1000 |
5 | >> | 1101 >> 2 | 0011 |
6 | ^ | 1010 ^ 0010 | 1000 |
C Online Test on Bitwise Operators
1 | C Programming Bitwise Operators Online Test 1 |